The Method Is Undefined for the Type Error in Java
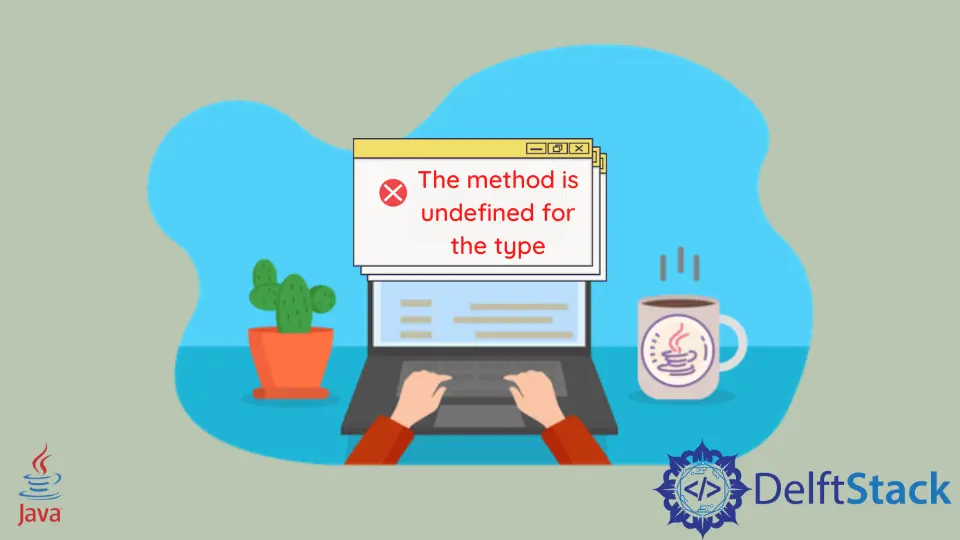
This tutorial demonstrates Java’s the method is undefined for the type
error.
the Method Is Undefined for the Type Error in Java
The error the method is undefined for the type
occurs whenever we try to call a method that is not defined in the current class. An example throws the the method is undefined for the type
error.
package delftstack;
public class Delftstack1 {
Delftstack1() {
System.out.println("Constructor of Delftstack1 class.");
}
static void delftstack1_method() {
System.out.println("method from Delftstack1");
}
public static void main(String[] args) {
delftstack1_method();
delftstack2_method();
}
}
class Delftstack2 {
Delftstack2() {
System.out.println("Constructor of Delftstack2 class.");
}
static void delftstack2_method() {
System.out.println("method from Delftstack2");
}
}
The code above calls a method from the class Delftstack2
in the class Delftstack1
directly without creating an instance of the object which will throw the error. See output:
Exception in thread "main" java.lang.Error: Unresolved compilation problem:
The method Delftstack2_method() is undefined for the type Delftstack1
at DelftstackDemos/delftstack.Delftstack1.main(Delftstack1.java:12)
To fix this problem, we have to instantiate the object of the Delftstack2
class in the Delftstack1
class. See the solution:
package delftstack;
public class Delftstack1 {
Delftstack1() {
System.out.println("Constructor of Delftstack1 class.");
}
static void delftstack1_method() {
System.out.println("method from Delftstack1");
}
public static void main(String[] args) {
delftstack1_method();
Delftstack2 delftstack2 = new Delftstack2();
delftstack2.delftstack2_method();
}
}
class Delftstack2 {
Delftstack2() {
System.out.println("Constructor of Delftstack2 class.");
}
static void delftstack2_method() {
System.out.println("method from Delftstack2");
}
}
The code above will work properly now. See output:
method from Delftstack1
Constructor of Delftstack2 class.
method from Delftstack2
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn FacebookRelated Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack