How to Use the System.exit() Method in Java
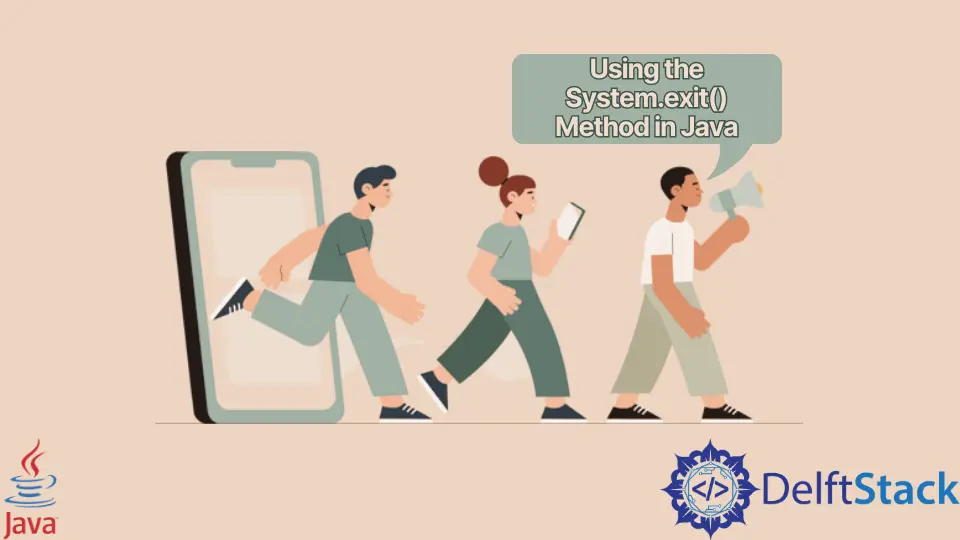
This tutorial introduces what the System.exit()
method does in Java.
System
is a class in Java that provides several utility methods to handle system-related tasks, such as the exit()
method used to stop the current execution and JVM and exit the control out to the program. We can use this method in our code to exit the current flow.
The general syntax of this method is given below.
public static void exit(int status)
It terminates the currently running Java Virtual Machine.
It takes a single integer argument which serves as a status code. By convention, a nonzero status code indicates abnormal termination.
This method calls the exit
method in class Runtime
. This method never returns normally. Internally it is similar to the below code.
Runtime.getRuntime().exit(n)
This method throws the SecurityException
if a security manager exists, and its checkExit
method doesn’t allow exit with the specified status.
The System.exit()
Method in Java
This example used the exit()
method to exit the program if any list element is greater than 50
. If elements are less than 50
, it prints the largest element, but if any element is greater than 50
, it exits and prints bye to the console.
See the example below.
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class SimpleTesting {
public static void main(String[] args) {
List<Integer> list = new ArrayList<>();
list.add(23);
list.add(32);
list.add(33);
System.out.println(list);
Integer result = getMax(list);
System.out.println("result " + result);
list.add(80);
result = getMax(list);
System.out.println("result " + result);
}
public static Integer getMax(List<Integer> list) {
if (Collections.max(list) > 50) {
System.out.println("Bye");
System.exit(1);
return Collections.max(list);
}
return Collections.max(list);
}
}
Output:
[23, 32, 33]
result 33
Bye