How to Create swing timer in Java
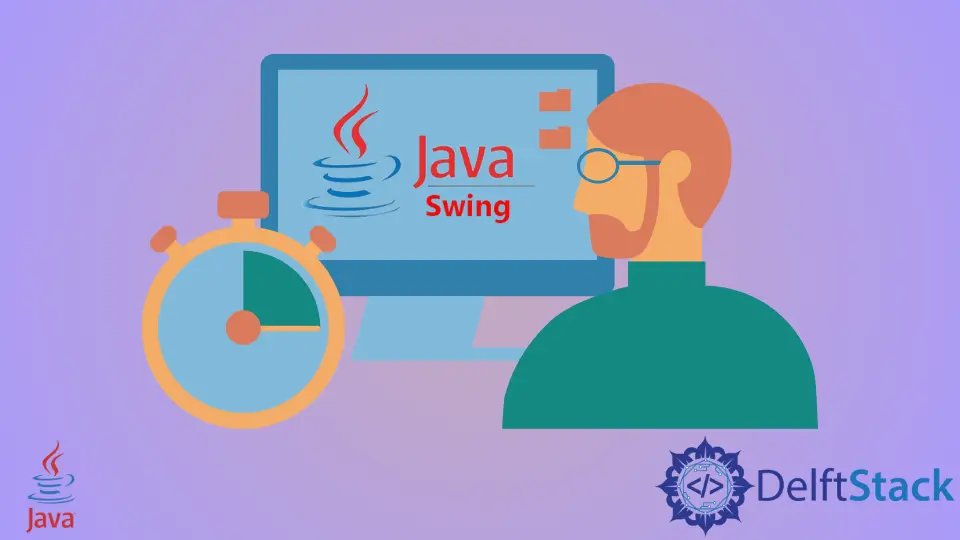
This tutorial introduces how to create and call a swing timer in Java.
Time
is a class in Swing used to execute tasks based on the specified interval.
To create a timer, we first need to create an action listener, then define the actionPerformed()
method in it to perform a task. After that, call the Timer()
constructor with all the arguments and call the start()
method to start the task.
The setRepeats()
method is used to call the actionPerformed()
repeatedly or only once as per the boolean argument. This method takes a boolean argument, either true or false and if you want to call the actionPerformed()
method repeatedly, then pass true, else pass false to it. Use timer.stop()
method to stop the task.
Let’s understand by some examples.
Create a Swing Timer in Java
In this example, we created a timer using the Timer
class and defined a task using the ActionListener
anonymous class with an actionPerformed()
method.
To start the timer, we used the start()
method and the stop()
method to stop the timer. The setRepeats()
method is used to start the task repeatedly.
The timer()
constructor takes two arguments: the delay time in milliseconds and an instance of action listener. See the example below.
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.Timer;
public class SimpleTesting {
public static void main(String[] args) throws InterruptedException {
ActionListener taskPerformer = new ActionListener() {
public void actionPerformed(ActionEvent evt) {
System.out.println("Timer is running");
}
};
Timer timer = new Timer(400, taskPerformer);
timer.setRepeats(true);
timer.start();
Thread.sleep(2500);
timer.stop();
}
}
Output:
Timer is running
Timer is running
Timer is running
Timer is running
Timer is running