super in Java
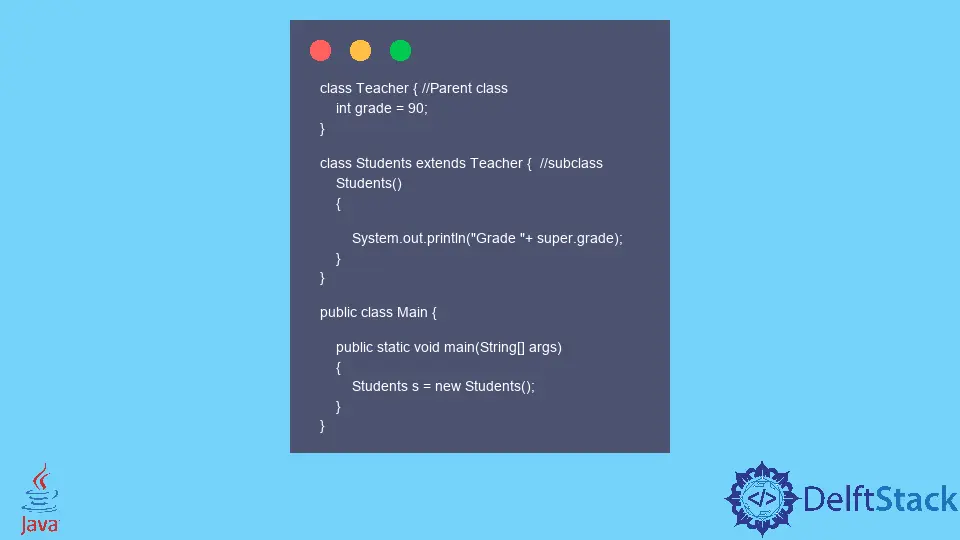
In Java, we have a predefined keyword super
that is somewhat related to the parent class. We will be discussing the super()
function in detail in this tutorial.
In Java, the super
keyword acts as a reference variable for the parent class objects. It can be used to call the parent’s class methods and variables.
For example
class Teacher { // Parent class
int grade = 90;
}
class Students extends Teacher { // subclass
Students() {
System.out.println("Grade " + super.grade);
}
}
public class Main {
public static void main(String[] args) {
Students s = new Students();
}
}
Output:
Grade 90
In the above example, we accessed the variable grade
from parent class Teacher
from the constructor of its subclass Students
using the super
keyword.
We also have the super()
function, which invokes the parent’s class constructor. This function is only used for calling the constructors of the parent class. Both parametric and non-parametric constructors can be called by using the super()
function. If somehow the constructor does not invoke a superclass constructor explicitly while using the super()
function, then the no-argument constructor of the superclass is called by the Java compiler itself.
See the code below.
class Teacher { // Parent class
Teacher() // constructor
{
System.out.println("Teacher class Constructor");
}
}
class Students extends Teacher { // subclass
Students() {
super();
System.out.println("Students class Constructor");
}
}
public class Main {
public static void main(String[] args) {
Students s = new Students();
}
}
Output:
Teacher class Constructor
Students class Constructor
We created a parent class Teacher
and a parent class constructor in the above code, which prints a message. Then a subclass Students
is extended from Teacher
, which has a constructor Students()
that consists of the super()
function for invoking the parent class constructor. Then we have the main class, which calls the subclass for executing the program. The super()
function invoked the parent class constructor to print the message from the parent class and then from the subclass.