StdDraw Java Import
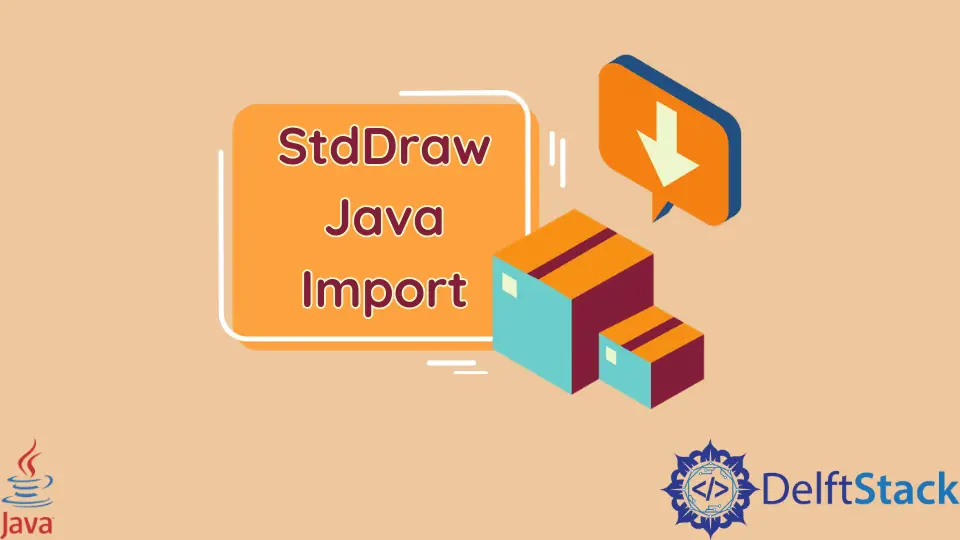
This tutorial demonstrates how to import the stddraw
in Java.
Import the StdDraw
Class in Java
The StdDraw
class is a part of the StdLib
package, which is used for the basic capabilities of drawing. This class allows us to draw simple drawings like lines, squares, circles, and other geometric shapes, including text, animations, and colors.
The StdDraw
class includes ActionListener
, KeyListener
, MouseListener
, MouseMotionListener
, and EventListener
interfaces which are used to perform events on different listener options.
As mentioned above, the StdDraw
is included in the StdLib
package, and the StdLib
package is included in the basic JRE or JDK package. If you can’t find the StdDraw
, you can always import it separately in your environment.
Follow the steps below to import the StdDraw
in your environment:
-
First, we must download the
stdlib.jar
file as theStdDraw
is included in that package. Download it from here: -
Create a folder
lib
in your Java project folder. -
Copy the downloaded
StdLib.jar
file to thelib
folder. -
Open your IDE; in our case, it is the
eclipse
. -
Right-click on your project name and go to
Properties
. -
In
Properties
, go toJava Build Path
: -
In Java Build Path, go to
Libraries
. -
Now, under the classpath, Click on
Add External Jar
, browse to theLib
folder, and selectstdlib.jar
. -
Once the jar is added to the classpath. Click
Apply and Close
, and you are ready to use theStdDraw
package.
Now we can use the StdDraw
class without importing it into our Java class file.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook