Difference Between Static and Non-Static Methods in Java
- Static Method in Java
- Non-Static Method in Java
- Difference Between Static and Non-Static Methods in Java
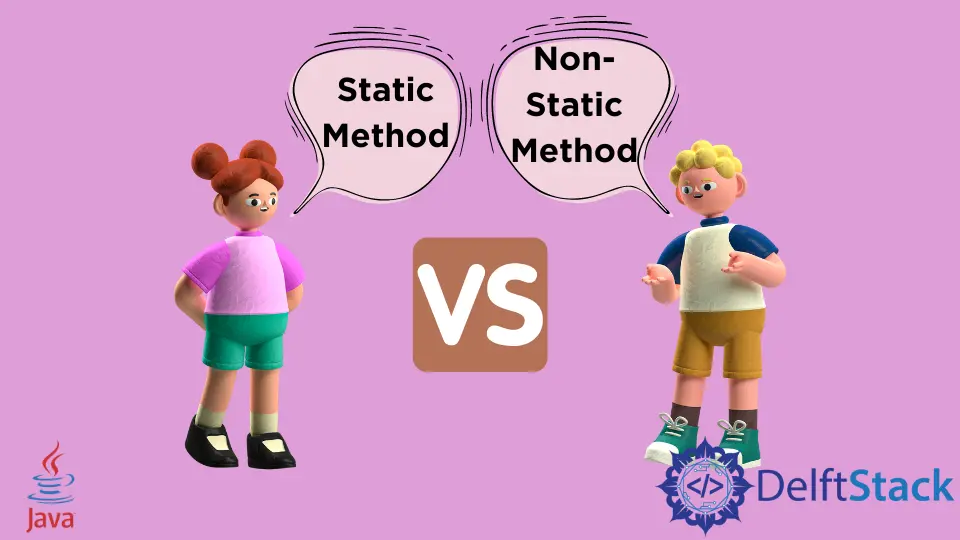
Static and non-static methods are elementary to comprehend. This article discusses their attributes, logical flow, and overall implementation.
Initially, we will run an example on the static method; then, we will show a simple demo on non-static. Finally, we will classify both in a table and use both in the single code block.
Static Method in Java
In Java, static
is a particular reserved keyword, which allows a method to function without a class instance.
Since a static
method is part of the class, we don’t need to create an instance. Let’s get to the point directly; check out the code below.
Code:
// This demo shows how you can use static methods!
public class StaticMethodDemo {
// We define static keyword to use static method
static void demoStaticMethod() {
System.out.println("We can call static methods without having to create their objects");
}
// Function
public static void main(String[] args) {
demoStaticMethod(); // This calls our static method
}
}
Output:
We can call static methods without having to create their objects
Non-Static Method in Java
The static
keyword is not necessary to name a non-static method or variable. It also corresponds to a class object; therefore, you’ll need to construct an instance of the class to get access to it.
Code:
class NonStaticMethodDemo {
public void NonStaticDemo() {
System.out.println("This is a demonstration of a Non Static Method in Java");
}
public static void main(String agrs[]) {
NonStaticMethodDemo NonSM = new NonStaticMethodDemo();
NonSM.NonStaticDemo();
}
}
Output:
This is a demonstration of a Non Static Method in Java
We don’t have to call the object to the static
method in the previous example.
On the contrary, you can not directly call a non-static method. It will throw a compilation error.
Check the difference out in the following example.
Difference Between Static and Non-Static Methods in Java
Static methods do not need instances variable; else, you will get a compilation error. In contrast, you can call non-static methods with the instance variable.
Difference Table:
Attributes | Static | Non-Static |
---|---|---|
Accessibility | Access only static methods | It can access both |
Declaration | We declare it with static keyword-only |
Does not require special keyword |
Memory | Less Memory (Allocation of memory occurs once) | More memory |
Binding | Uses compile-time binding | Run time-binding/Dynamic binding |
Syntax | static void DemoStatic() {} |
void IamNonStaticDemo() {} |
Steps of Implementation for a Code with Static and Non-Static Methods in Java
- Class -
public class DifferenceBtwStaticVsNonStaticDemo {}
- Static vs Non-Static Variable -
String demo = "Variable";
static String var = "Static Varibale";
- Instance -
DifferenceBtwStaticVsNonStaticDemo instance = new DifferenceBtwStaticVsNonStaticDemo();
. We will use this instance to callIamNonStaticDemo
method. - Static Method does not need an instance -
DifferenceBtwStaticVsNonStaticDemo.DemoStatic();
Now, check the following program.
Code:
public class DifferenceBtwStaticVsNonStaticDemo { // main class
// we will call these variables in both methods
String demo = "Variable";
static String var = "Static Variable";
// main function
public static void main(String[] args) {
// Your code goes here
// Creating Instance of the main class
DifferenceBtwStaticVsNonStaticDemo instance = new DifferenceBtwStaticVsNonStaticDemo();
// instance will call a non static method
instance.IamNonStaticDemo();
// Static methods do not need instance variables
DifferenceBtwStaticVsNonStaticDemo.DemoStatic();
}
// This is static method
static void DemoStatic() {
System.out.println("Static Method:::::" + var); // static variable for static method
}
// This is non static method
void IamNonStaticDemo() {
System.out.println("Non Static Method:::::" + demo + " I can also access:::: "
+ var); // Since it is a non static method, it can access both string variables
}
} // main class
Output:
Non Static Method:::::Variable I can also access:::: Static Variable
Static Method:::::Static Variable
Sarwan Soomro is a freelance software engineer and an expert technical writer who loves writing and coding. He has 5 years of web development and 3 years of professional writing experience, and an MSs in computer science. In addition, he has numerous professional qualifications in the cloud, database, desktop, and online technologies. And has developed multi-technology programming guides for beginners and published many tech articles.
LinkedIn