Static VS Non-Static Enum in Java
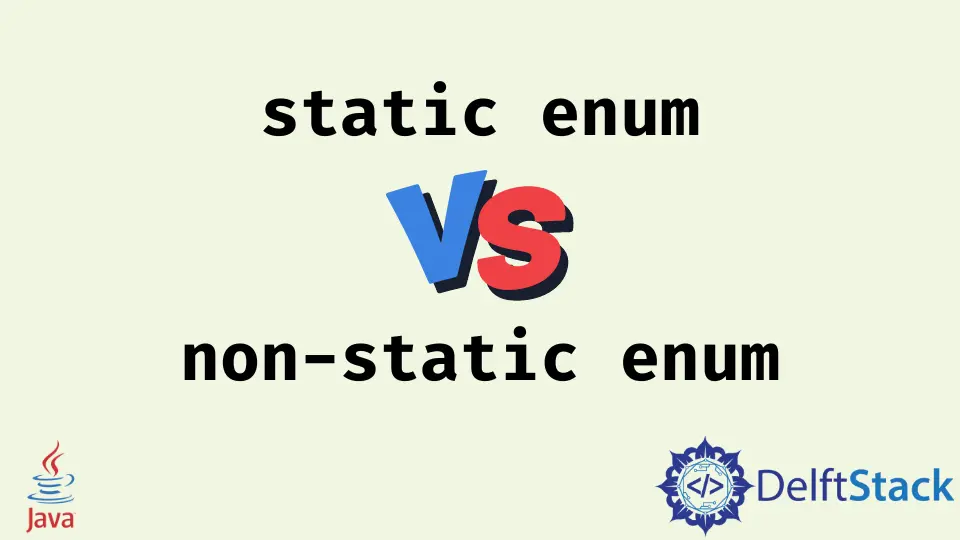
This article introduces enum
in Java and the difference between static and non-static enums
.
Introduction to Enum
in Java
In Java, an enum
is a data type that a programmer can use to create multiple constant variables. Generally, the programmer uses the final
keyword to declare the constant variable in Java, but programmers can declare all the final variables at a time and assign their initial value using an enum
.
Syntax to use Enum
:
public enum EnumA { En1, En2 }
public class demo {
public static void main(String[] args) {
EnumA test = EnumA.En1;
}
}
In the above syntax, users can see that EnumA
contains the En1
and En2
constant variables and how we have accessed them inside the static method of the demo
class.
Static VS Non-Static Enum
in Java
Now, we will differentiate the Enum
declared with the static
and without the static
keyword. Users can look at the code below to learn to declare the Enum
with and without the static
keyword.
Syntax of Enum
With and Without Static
Keyword:
public class demo {
public static enum EnumA { En1, En2 }
public enum EnumB { En3, En4 }
public static void main(String[] args) {
EnumA test1 = EnumA.En1;
EnumB test2 = EnumB.En3;
}
}
In the above syntax, users can see that EnumA
is defined with the static
keyword and EnumB
without the static
keyword. However, we are accessing both Enum
’s constants in the same way, concluding that in Java, all Enum
is static even if users use the static
keyword while defining it.
In Java, we can only access the static members and execute static methods of a class without an object reference inside the public static
method.
Furthermore, developers can observe that we are accessing the variables of EnumA
and EnumB
inside the public static
method without referencing an object of its class which means Enum
declared without a static
keyword is also static by default.
So, the article concludes that even if programmers do not use the static
keyword while defining the Enum
, it is static by default.