How to Get the Square of a Double Value in Java
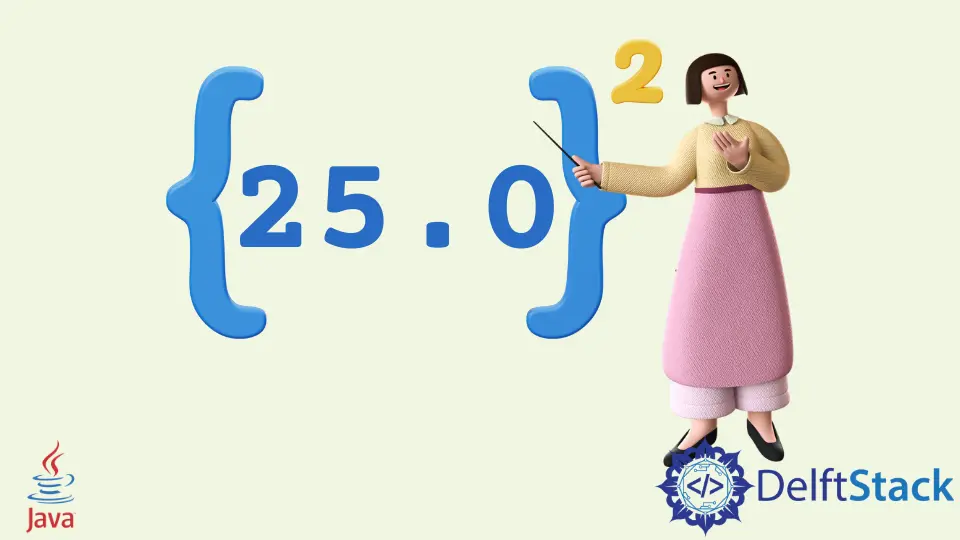
This tutorial introduces the ways to get the square of a double value in Java.
The square of a number is a product of the number (itself). For example, a square of 2 is 4 (2*2
). In Java, we can use several ways to get the square of any number, such as a built-in method of the Math
package or custom code.
This article will teach us to get the square of a double value in Java by using either a built-in method or our custom code. Let’s start with some examples.
Get Square by Multiplying in Java
In this example, we used the multiply operator to get the square of a double value. This is one of the easiest and fast ways to get the square of any number. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
double dval = 25.0;
System.out.println("double value = " + dval);
double result = dval * dval;
System.out.println("square result " + result);
}
}
Output:
double value = 25.0
square result 625.0
Get Square by Math.pow()
Method in Java
Java provides a built-in math method, pow()
, to get the square of any value. This method takes two arguments, one is the value, and the second is the power. We pass the second argument as 2 because we want a square as a result. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
double dval = 25.0;
System.out.println("double value = " + dval);
double result = Math.pow(dval, 2);
System.out.println("square result " + result);
}
}
Output:
double value = 25.0
square result 625.0