How to Covert Set to ArrayList in Java
- Understanding the Basics of Set and ArrayList
- Method 1: Using the Constructor of ArrayList
-
Method 2: Using the
addAll()
Method - Method 3: Using Streams (Java 8 and Above)
- Conclusion
- FAQ
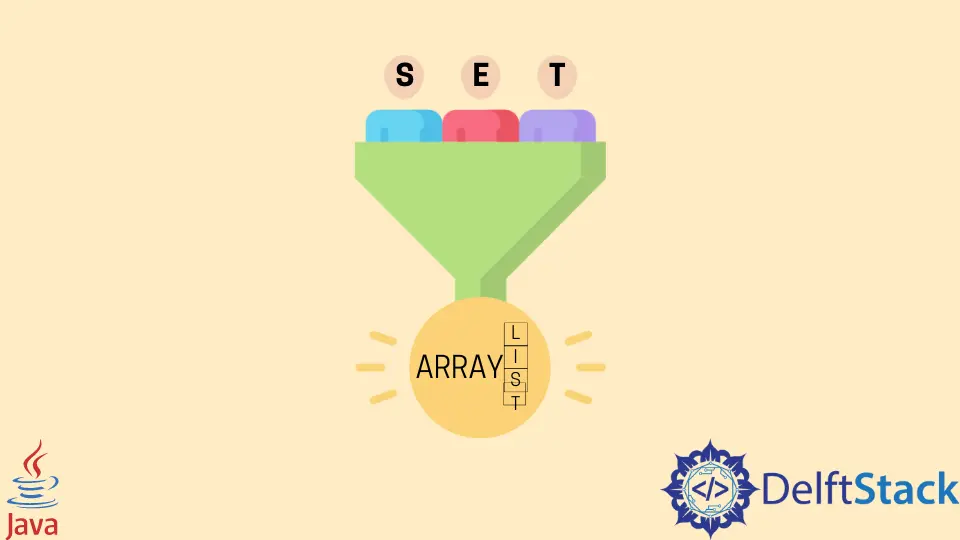
Converting a Set to an ArrayList in Java is a common task that many developers encounter. Whether you’re working with a HashSet, TreeSet, or any other Set implementation, the need to switch to an ArrayList arises when you want to take advantage of the ArrayList’s dynamic array capabilities.
In this article, we’ll explore various methods to achieve this conversion, along with clear examples and explanations. By the end, you’ll have a solid understanding of how to efficiently convert a Set to an ArrayList, enhancing your Java skills and making your code more versatile.
Understanding the Basics of Set and ArrayList
Before diving into the conversion methods, let’s briefly understand the two data structures involved. A Set in Java is a collection that does not allow duplicate elements and does not guarantee the order of elements. On the other hand, an ArrayList is a resizable array implementation of the List interface, allowing for indexed access and dynamic resizing.
The conversion process is straightforward, and Java’s Collections framework provides several ways to facilitate this. Let’s explore these methods in detail.
Method 1: Using the Constructor of ArrayList
One of the simplest ways to convert a Set to an ArrayList is by utilizing the ArrayList constructor that accepts a Collection. This method is efficient and concise, making it a popular choice among developers.
Here’s how you can do it:
import java.util.ArrayList;
import java.util.HashSet;
import java.util.Set;
public class SetToArrayList {
public static void main(String[] args) {
Set<String> set = new HashSet<>();
set.add("Apple");
set.add("Banana");
set.add("Cherry");
ArrayList<String> arrayList = new ArrayList<>(set);
System.out.println(arrayList);
}
}
Output:
[Banana, Cherry, Apple]
In this example, we first create a HashSet containing three fruit names. We then instantiate an ArrayList using the set as an argument. The ArrayList constructor automatically copies the elements from the Set. The output shows that the elements are now stored in an ArrayList, although the order may vary since Sets do not guarantee order.
This method is particularly efficient because it leverages the built-in capabilities of Java’s Collections framework, allowing for a clean and readable approach to conversion.
Method 2: Using the addAll()
Method
Another effective method to convert a Set to an ArrayList is by using the addAll()
method. This approach gives you more flexibility, as you can start with an empty ArrayList and then add elements from the Set.
Here’s how you can implement this:
import java.util.ArrayList;
import java.util.HashSet;
import java.util.Set;
public class SetToArrayList {
public static void main(String[] args) {
Set<String> set = new HashSet<>();
set.add("Dog");
set.add("Cat");
set.add("Rabbit");
ArrayList<String> arrayList = new ArrayList<>();
arrayList.addAll(set);
System.out.println(arrayList);
}
}
Output:
[Cat, Dog, Rabbit]
In this code snippet, we again create a HashSet with three animal names. We then create an empty ArrayList and use the addAll()
method to populate it with elements from the Set. The output reflects the contents of the ArrayList, which again may appear in a different order due to the nature of Sets.
This method is particularly useful when you want to initialize an ArrayList and populate it conditionally or in stages, as you can add elements from multiple collections if needed.
Method 3: Using Streams (Java 8 and Above)
If you are using Java 8 or later, you can take advantage of the Stream API to convert a Set to an ArrayList in a more functional style. This method is not only elegant but also allows for additional operations like filtering and mapping.
Here’s an example:
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import java.util.stream.Collectors;
public class SetToArrayList {
public static void main(String[] args) {
Set<String> set = new HashSet<>();
set.add("Red");
set.add("Green");
set.add("Blue");
List<String> arrayList = set.stream().collect(Collectors.toList());
System.out.println(arrayList);
}
}
Output:
[Red, Green, Blue]
In this example, we create a HashSet of color names and convert it to an ArrayList using the Stream API. The stream()
method generates a sequential Stream of the elements, and collect(Collectors.toList())
gathers them into a List. The output shows the ArrayList containing the colors.
This method is particularly powerful because it allows for more complex data transformations and operations during the conversion process, making your code more expressive and concise.
Conclusion
Converting a Set to an ArrayList in Java is a straightforward task that can be accomplished in several ways. Whether you choose to use the ArrayList constructor, the addAll()
method, or the Stream API, each method has its advantages and use cases. Understanding these methods will not only enhance your coding skills but also improve the efficiency and readability of your Java applications. So, the next time you need to perform this conversion, you’ll have the tools and knowledge to do it effectively.
FAQ
-
What is the difference between Set and ArrayList in Java?
Set does not allow duplicate elements and does not guarantee order, whereas ArrayList allows duplicates and maintains the order of insertion. -
Can I convert a TreeSet to an ArrayList?
Yes, you can convert a TreeSet to an ArrayList using the same methods described in this article. -
What happens to the order of elements when converting a Set to an ArrayList?
The order of elements in the ArrayList may differ from the original Set, as Sets do not guarantee any specific order. -
Is it possible to convert a Set to an ArrayList without using any Java Collections methods?
While you can manually iterate through the Set and add elements to an ArrayList, it is not recommended due to the extra complexity involved. -
Can I convert a Set of custom objects to an ArrayList?
Yes, you can convert a Set of custom objects to an ArrayList using the same methods, as long as the custom objects properly implement the required methods likeequals()
andhashCode()
.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedInRelated Article - Java Set
- How to Create a Concurrent Set in Java
- Union and Intersection of Two Java Sets
- How to Convert ArrayList to Set in Java
- How to Sort a Set in Java
- How to Find a Set Intersection in Java