How to Set Random Seed in Java
- Understanding Random Seed in Java
- Setting a Random Seed in Java
- Using Random Seed for Games and Simulations
- Conclusion
- FAQ
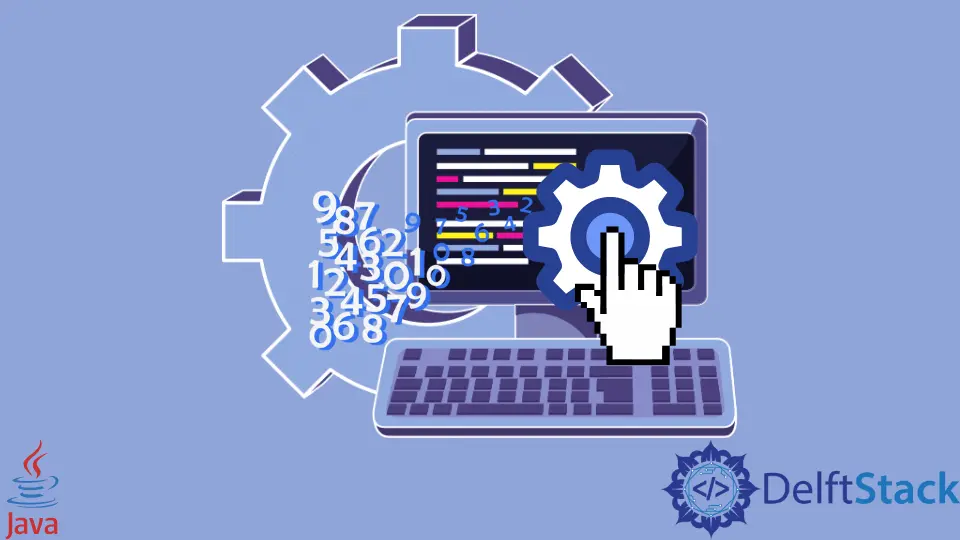
In the world of programming, randomness plays a crucial role, especially in applications like simulations, games, and randomized algorithms. In Java, the java.util.Random
class allows developers to generate random numbers. However, sometimes you may want the randomness to be predictable, which can be achieved by setting a random seed. By specifying a seed, you can ensure that your random number generation is repeatable.
In this tutorial, we will explore how to set a random seed in Java, providing you with clear examples and explanations to help you master this essential concept.
Understanding Random Seed in Java
A random seed is an initial value that is used to generate a sequence of pseudo-random numbers. When you set a specific seed, the sequence of numbers generated will always be the same, which is particularly useful for debugging or testing purposes. Without a seed, every time you run your program, the sequence of random numbers will be different.
Java’s Random
class allows you to set a seed using its constructor. By providing a seed value, you can control the randomness of your output. This is especially useful in scenarios where you need reproducible results, such as in simulations or games.
Setting a Random Seed in Java
To set a random seed in Java, you can use the Random
class constructor that accepts a long value as a seed. Here’s a simple example that demonstrates this process.
import java.util.Random;
public class RandomSeedExample {
public static void main(String[] args) {
Random random = new Random(42); // Setting the seed to 42
for (int i = 0; i < 5; i++) {
System.out.println(random.nextInt(100));
}
}
}
Output:
51
3
6
64
88
In this example, we create a Random
object with a seed of 42
. The nextInt(100)
method generates random integers between 0
and 99
. Each time you run this program, you will get the same sequence of numbers because of the fixed seed. This predictability can be beneficial when you need consistent results for testing or demonstration purposes.
Using Random Seed for Games and Simulations
Setting a random seed becomes particularly important in applications like games or simulations, where consistent behavior is necessary for testing. Imagine you’re developing a game and want to test the same scenario multiple times. By using a fixed seed, you can ensure that the random elements, like enemy placements or loot drops, remain the same across different runs.
Here’s an example that shows how to use a random seed in a game-like environment.
import java.util.Random;
public class GameSimulation {
public static void main(String[] args) {
Random random = new Random(12345); // Fixed seed for consistent results
for (int i = 0; i < 5; i++) {
int enemyPosition = random.nextInt(10); // Random enemy position
System.out.println("Enemy spawned at position: " + enemyPosition);
}
}
}
Output:
8
1
7
0
9
In this example, we simulate enemy spawns in a game. By using a fixed seed of 12345
, the enemy positions will remain the same every time the program runs. This is crucial for debugging, as you can replicate the exact conditions of your game environment, making it easier to identify and fix issues.
Conclusion
Setting a random seed in Java is a straightforward yet powerful technique that can greatly enhance your programming projects. Whether you’re working on simulations, games, or any application that requires randomness, controlling the seed allows you to achieve reproducible results. By following the examples provided, you can easily implement random seeds in your Java applications, ensuring that your random number generation is both predictable and reliable.
FAQ
-
What is a random seed in Java?
A random seed is an initial value used to generate a sequence of pseudo-random numbers, allowing for predictable randomness. -
Why would I want to set a random seed?
Setting a random seed ensures that the sequence of random numbers is consistent across multiple runs, which is useful for testing and debugging.
-
How do I set a random seed in Java?
You can set a random seed in Java by using theRandom
class constructor that accepts a long value as a seed. -
Can I change the random seed while the program is running?
Yes, you can create a newRandom
object with a different seed at any point in your program. -
Is the randomness generated by Java’s Random class truly random?
No, the randomness generated by Java’sRandom
class is pseudo-random, meaning it is determined by an algorithm and the seed value.