How to Run Java Programs From Command Line
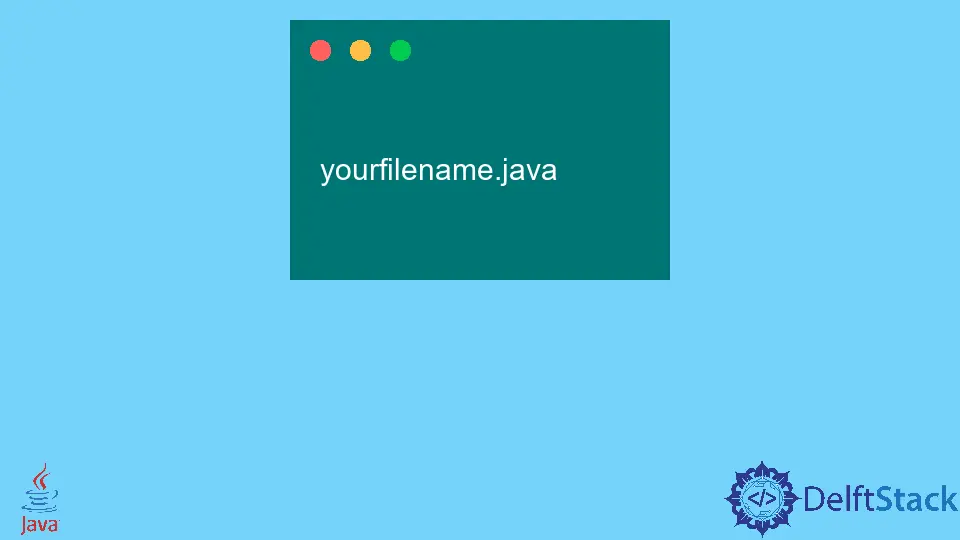
Java programming is very flexible and robust. We can run its programs from the command line also. For this, Java must be installed on your computer before you can proceed.
We will discuss in this tutorial on how to run Java Programs from command line.
First, we need the bin directory from where JDK is installed. Generally, it can be found in the following path.
"C:\Program Files\Java\jdk1.8.0_91\bin"
Now we open the command prompt and go to the directory containing the file we need to run. Here we need to set the path so that the system can find the necessary JDK programs and tools.
set path=%path%;C:\Program Files\Java\jdk1.5.0_09\bin
Now, ensure that your Java file should be like this with the extension .java
.
yourfilename.java
We first compile the .java
file to a .class
file using the javac
command.
javac yourfilename.java
Finally, we can run the file. The result is displayed on the command prompt window.
java yourfilename
If any error occurs while running the javac
command, it may be because the path is not set correctly.
We can also run .jar
files using the command line. For this, we need to make the executable file that has the extension .jar
.
There are two ways to do this. In the first method, we can use the exterior manifest file. The manifest file is just an external entry of the main class.
jar -cvfm yourfilename.jar manifest.mf yourfilename.class
Alternatively, we can also use the entry point as shown below.
jar -cvfm yourfilename.jar <MainClass> yourfilename.class
After this, we can run the executable file. For this, we can run the following command.
java -jar yourfilename.jar