Roman Numerals in Java
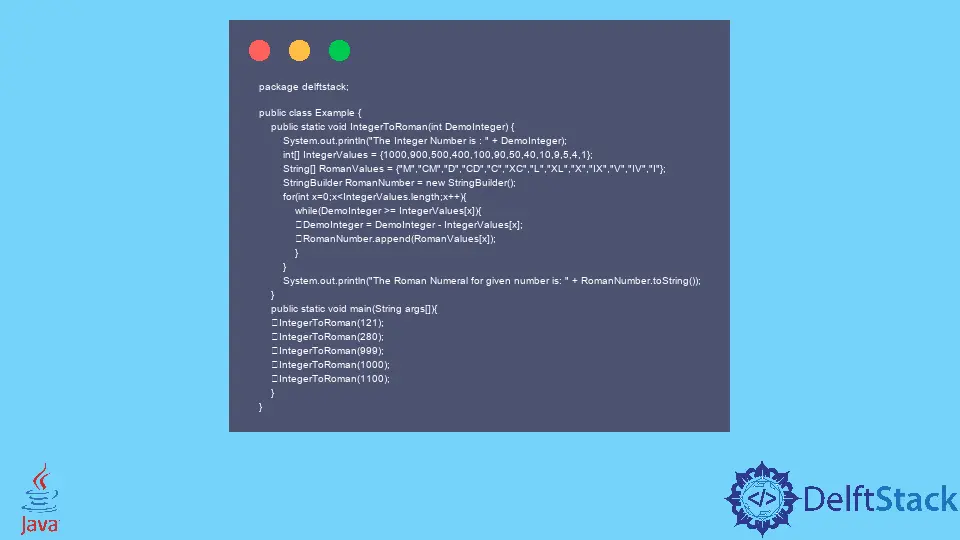
This tutorial demonstrates how to convert integers to roman numerals in Java.
Roman Numerals in Java
Converting the integers to roman numerals is frequently required while working in Java. Most of the time, this question is asked during interviews with the TOP IT companies.
Roman numerals are the character representation of numbers; we can usually find these numbers in watches or music theory. There are a total of seven letters that are used to represent a number in roman characters; see the table below:
Roman | Numbers |
---|---|
I | 1 |
IV | 4 |
V | 5 |
IX | 9 |
X | 10 |
XL | 40 |
L | 50 |
XC | 90 |
C | 100 |
CD | 400 |
D | 500 |
CM | 900 |
M | 1000 |
The roman numerals are usually written from left to right and highest to lowest. The above table shows that we can not use a roman numeral more than three times; for example, we will write 4 like IV but not IIII.
Now let’s try to create an approach in Java to convert integers to roman numerals. Follow the steps below:
-
First, we have to create two arrays, one for the roman numeral and one for integers.
-
Then, create an instance of the string builder class.
-
The next step is to compare the integer with roman numerals.
-
If the integer is
>=
, then the highest roman numeral, we need to add it to the string builder instance and reduce its value from the input number. -
If the integer is
<
, then the highest roman numeral, we need to check to the highest roman numeral and repeat the process until the input number is 0. -
The final string builder will be the converted roman numeral.
Now let’s try to implement a Java program based on the above steps:
package delftstack;
public class Example {
public static void IntegerToRoman(int DemoInteger) {
System.out.println("The Integer Number is : " + DemoInteger);
int[] IntegerValues = {1000, 900, 500, 400, 100, 90, 50, 40, 10, 9, 5, 4, 1};
String[] RomanValues = {"M", "CM", "D", "CD", "C", "XC", "L", "XL", "X", "IX", "V", "IV", "I"};
StringBuilder RomanNumber = new StringBuilder();
for (int x = 0; x < IntegerValues.length; x++) {
while (DemoInteger >= IntegerValues[x]) {
DemoInteger = DemoInteger - IntegerValues[x];
RomanNumber.append(RomanValues[x]);
}
}
System.out.println("The Roman Numeral for given number is: " + RomanNumber.toString());
}
public static void main(String args[]) {
IntegerToRoman(121);
IntegerToRoman(280);
IntegerToRoman(999);
IntegerToRoman(1000);
IntegerToRoman(1100);
}
}