How to Re-Throw Exception in Java
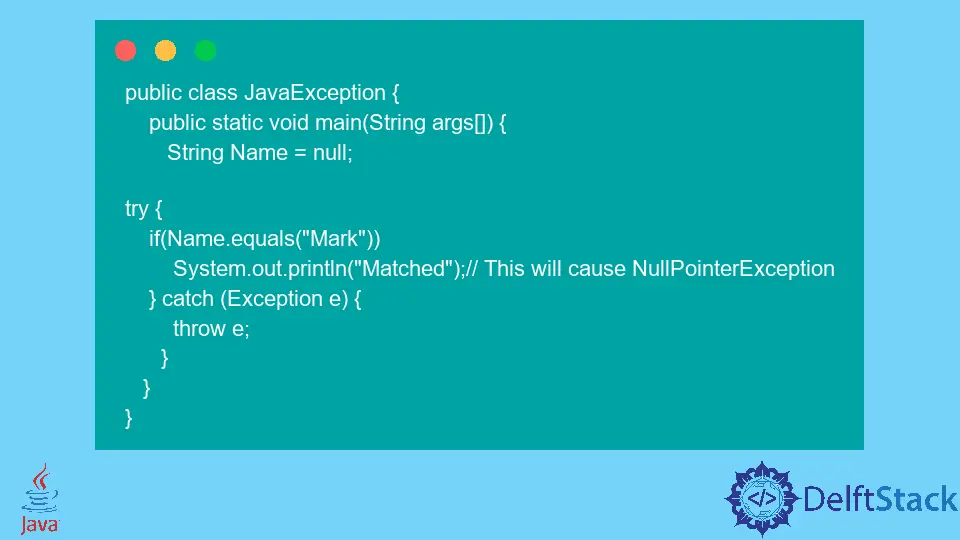
In Java, throw
is the most commonly used keyword to throw either a built-in
exception or a -user-defined
exception, but sometimes we have to throw
the same exception through the catch
block.
This situation is called the re-throwing an exception. In this tutorial article, we will learn about the re-throwing exception and perform this task without making any changes to the original stack trace.
Demonstration of Re-Throwing an Exception in Java
If you are working with sensitive programs, such as manipulating the database, tracking the exception is essential before propagating it to a higher level.
We need to perform some activities on the catch
block and re-throw the exception so that the higher level of the program gets notified that an exception occurs in the system.
In our example below, we will re-throw an exception when a string
value sets to null
. The code for this purpose will be like the below:
public class JavaException {
public static void main(String args[]) {
String Name = null;
try {
if (Name.equals("Mark"))
System.out.println("Matched"); // This will cause NullPointerException
} catch (Exception e) {
throw e;
}
}
}
In the above code fence, we first set the value of a string
variable Name
to null
. After that, we used the exception handler of java try{ ... } catch { ... }
, and inside it, we compared the string
value with another string
.
Now this will cause a NullPointerException
error. After running the above example code, you will get the below output in your console.
Exception in thread "main" java.lang.NullPointerException
at javacodes.JavaException.main(JavaException.java:8)
If we look at the code of the example sincerely, we will see that our code re-throws any exception is caught, and we get the original stack trace without making any changes.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn