How to Resolve java.io.IOException: Broken Pipe
-
Understand the
java.io.IOException: Broken pipe
-
Causes of
java.io.IOException: Broken pipe
-
Solution for the
java.io.IOException: Broken pipe
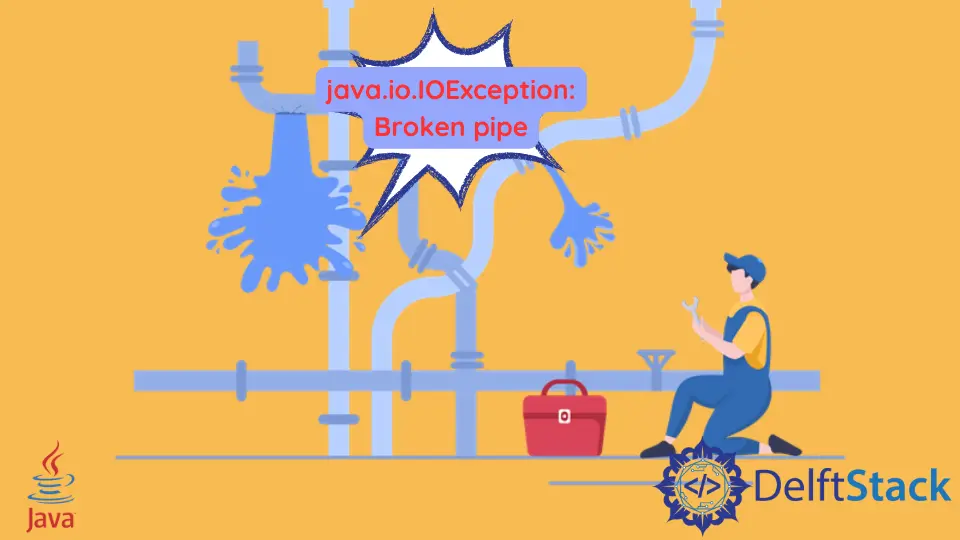
This article covers the causes of the java.io.IOException: Broken pipe
exception and provides solutions to fix it in Java. But before that, let’s look at the java.io.IOException: Broken pipe
exception.
Understand the java.io.IOException: Broken pipe
java.io.IOException: Broken pipe
refers to the situation in which one device is trying to read/write data from/to a pipe while the machine previously connected to the other end of the pipe has either died or been terminated.
We must establish a new connection to continue data transmission because that connection has been severed. If we don’t do it, it will terminate the data transfer, and we will receive the following exception:
java.io.IOException : Broken pipe
Causes of java.io.IOException: Broken pipe
Following are some potential causes that trigger the java.io.IOException: Broken pipe
:
- When a client terminates an open connection while doing any operation, such as loading a page or downloading a file. It is one of the most common things that can cause this exception.
- Another common reason for a
java.io.IOException: Broken pipe
is when one of two machines interacting via a socket has shut down the socket on its end before the exchange of information has been finished. - Performance difficulties or low network efficiency can disrupt a connection between a client and a server and lead to exceptions.
- When the web server cannot get a response from the service within a specified amount of time, which is equal to the timeout value defined in the server, it will shut the connection on the client end, resulting in a
Broken Pipe
.
Solution for the java.io.IOException: Broken pipe
- We can try inserting some delays throughout the application to see if this makes a difference. However, because of the delays, the users should have sufficient time to complete the transfer.
- Handle the exception dignifiedly by performing the necessary logging or taking action.
- To be prepared for any situation, we should improve your internet connection as soon as possible.
- Increasing the Timeout of the Server, which has a default value of 60 seconds. Increasing this amount will not only reduce the likelihood of a
Broken Pipe
, but it will also improve performance.
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedInRelated Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack