How to Replace Character in String in Java
-
String.replace()
to Replace a Single Character in a Java String -
String.replaceFirst()
to Replace Only the First Occurrence of a Character in a Java String -
String.replace()
to Replace Two Characters in a String in Java
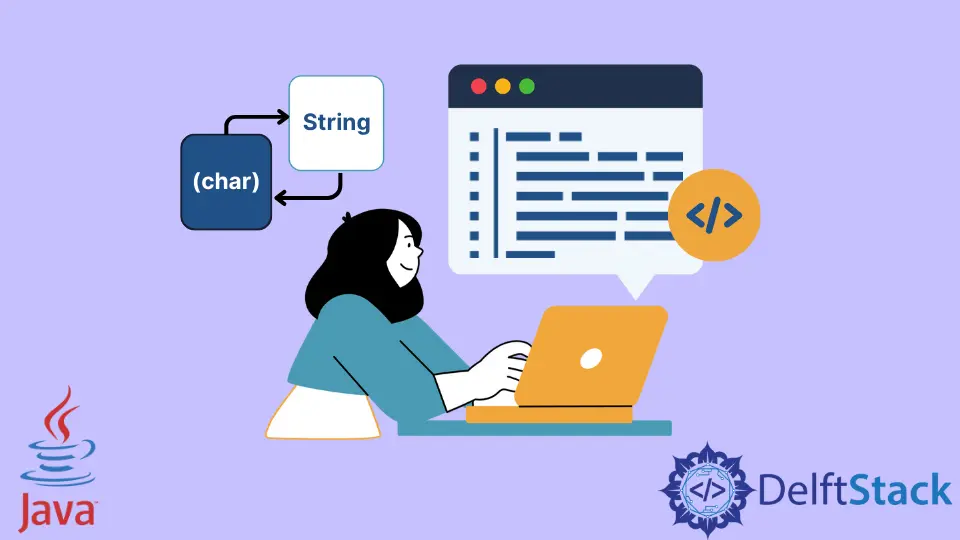
In this tutorial, we will introduce two methods, replace()
and replaceFirst()
of the String
class, replacing one or more characters in a given string in Java.
String.replace()
to Replace a Single Character in a Java String
We can use the replace()
method to replace a single character in a string. replace(oldChar, newChar)
demands two arguments: the first argument is the character that we want to be replaced, and the second argument is a new character that is to replace the old character.
In the following example, we have a string oldString1
containing a statement with an &
, but we want to replace it with a coma. This can be simply done by calling the replace()
method using oldString1
and passing &
and a coma.
One important thing to notice here is that there is whitespace before &
in replace()
. It is because there is whitespace around our target character. To eliminate the whitespace, we will replace both &
and the whitespace with coma.
public class ReplaceCharString {
public static void main(String[] args) {
String oldString1 = "My name is Sam & I am a software developer.";
String newString1 = oldString1.replace(" &", ",");
System.out.println(newString1);
}
}
Output:
My name is Sam, I am a software developer.
String.replaceFirst()
to Replace Only the First Occurrence of a Character in a Java String
There might be more than one occurrence of the same character we want to replace in a string. If we want to replace only the character’s first occurrence and ignore other occurrences after that. It can be done using another method of the String
class, which is replaceFirst()
. As its name suggests, it replaces only the first character of a string.
In the example, we have a string with two &
, and we only want to replace the first one with a coma as we did in the previous example, but ignore the second &
. So, we use oldString.replaceFirst(oldChar, newChar)
to pass whitespace with &
and a coma. The output shows the final result.
public class ReplaceCharString {
public static void main(String[] args) {
String oldString1 =
"I have used multiple Internet providers & but my current provider is AT&T.";
String newString1 = oldString1.replaceFirst(" &", ",");
System.out.println(newString1);
}
}
Output:
I have used multiple Internet providerss, but my current provider is AT&T.
String.replace()
to Replace Two Characters in a String in Java
In the last example of this tutorial, we will use replace()
to replace two different characters. In oldString1
, we want to replace the capital letter character (V
) with a small letter character (v
), and the last character of the string, coma ,
with .
.
We can do this in a single line by joining two replace()
methods and then passing the proper characters.
public class ReplaceCharString {
public static void main(String[] args) {
String oldString1 = "My name is Sam and I am a Software DeVeloper,";
String newString1 = oldString1.replace("V", "v").replace(",", ".");
System.out.println(newString1);
}
}
Output:
My name is Sam and I am a Software Developer.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedInRelated Article - Java String
- How to Perform String to String Array Conversion in Java
- How to Remove Substring From String in Java
- How to Convert Byte Array in Hex String in Java
- How to Convert Java String Into Byte
- How to Generate Random String in Java
- The Swap Method in Java