How to Replace a Backslash With a Double Backslash in Java
-
Replacing a Single Backslash (
\
) With a Double Backslash (\\
) in Java -
Replacing a Single Backslash(
\
) With a Double Backslash(\\
) Using thereplaceAll()
Method
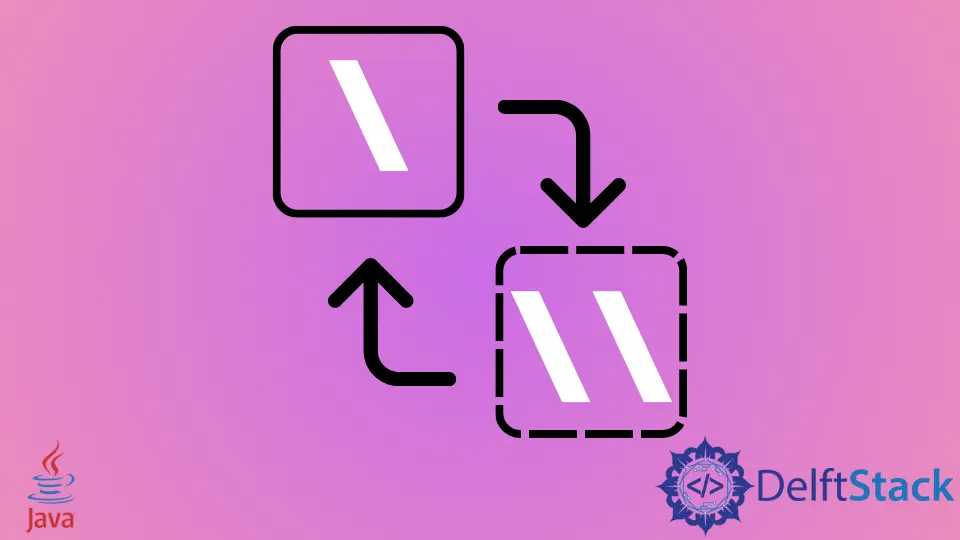
This tutorial introduces how to replace a single backslash (\
) with a double backslash (\\
) in Java.
The single and double backslashes are used to form a path of file or folder in an operating system. Java also follows these styles to locate any resource in the memory. Java String uses doubled backslashes due to conventions. In this article, we will learn to replace a single backslash with double backslashes. Let’s start with some examples.
Replacing a Single Backslash (\
) With a Double Backslash (\\
) in Java
In this example, we used the replace()
method of the String
class to replace the single backslash with a double backslash. This method returns a new modified String object. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
String msg = "Hello \\ my name is delft";
System.out.println(msg);
String newmsg = msg.replace("\\", "\\\\");
System.out.println(newmsg);
}
}
Output:
Hello \ my name is delft
Hello \\ my name is delft
Replacing a Single Backslash(\
) With a Double Backslash(\\
) Using the replaceAll()
Method
This is another solution that you can use to replace the backslashes. Here, we used the replaceAll()
method that works fine and returns a new String object. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
String msg = "Hello \\ my name is delft and you visit \\ @ delftstack.com";
System.out.println(msg);
String newmsg = msg.replaceAll("\\\\", "\\\\\\\\");
System.out.println(newmsg);
}
}
Output:
Hello \ my name is delft and you visit \ @ delftstack.com
Hello \\ my name is delft and you visit \\ @ delftstack.com