How to Read Integers From a File in Java
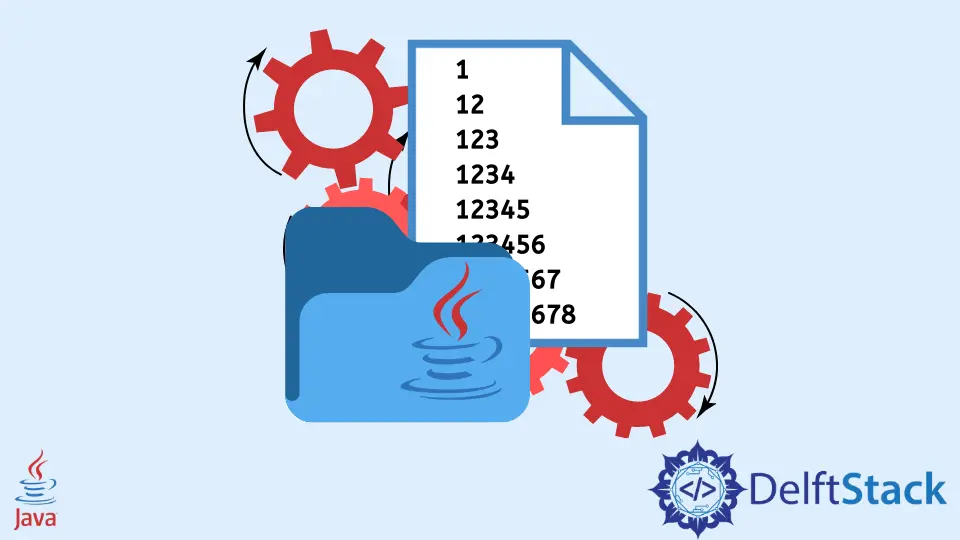
To read integers from a file, we use BufferedReader
to read the file and the parseInt()
method to get the integers from the data. This tutorial demonstrates a step-by-step process of how to read integers from a file in Java.
Read Integers From a File in Java
The BufferedReader
class is used to perform the read and stream of characters from the files or other sources in Java. The BufferedReader
class has a method readline()
, which reads every next line and returns a string.
The class BufferedReader
doesn’t provide any method to read the integer from a file, so initially, we read integers as strings, then we use the method parseInt()
to parse the string into an integer. The step-by-step process of the whole method is given below.
-
First, use the
BufferedReader
andFileReader
to read the text file. -
Now, create a
while
loop to read each line. The loop will read the file until the next entry isnull
. -
Then, read the integer values as a string using the
readLine()
method. -
Finally, parse the string into integers using the
parseInt()
method from theinteger
class and use or print them.
Let’s implement the steps in Java code.
package delftstack;
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class Read_Integer {
public static void main(String args[]) throws IOException {
BufferedReader reader = new BufferedReader(new FileReader("delftstack.txt"));
String Int_line;
while ((Int_line = reader.readLine()) != null) {
int In_Value = Integer.parseInt(Int_line);
// Print the Integer
System.out.println(Int_line);
}
}
}
The file we are trying to read is:
The output for the code will be:
1
12
123
1234
12345
123456
1234567
12345678
123456789
1234567890
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook