How to Use of the flush() Method in Java Streams
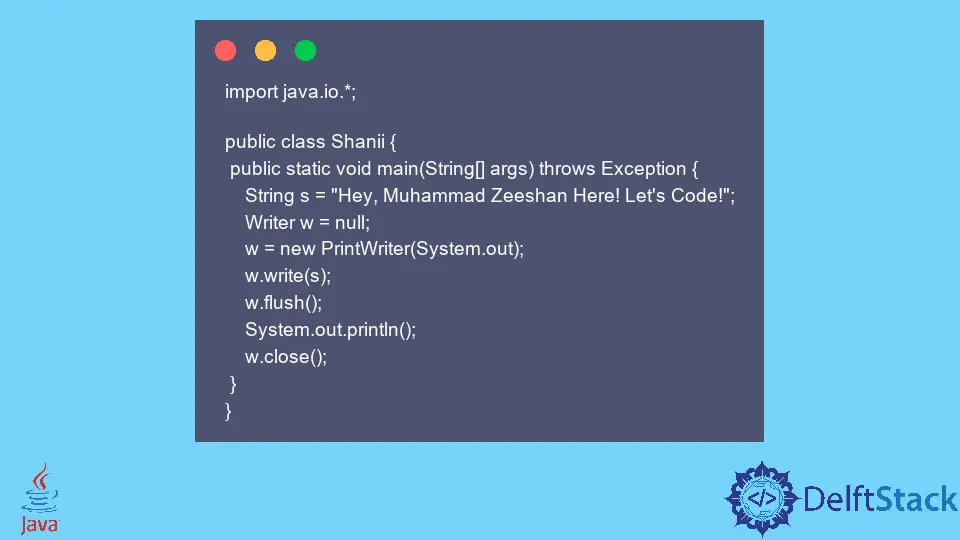
This article will discuss the function flush()
plays in Java streams and its primary purpose.
the flush()
Method in Java
This Writer stream may be flushed using the flush()
function, which can be found in the Writer
class. After determining whether or not the destination held another character or byte stream, the destination was flushed if the stream saved any characters from the various write()
methods in a buffer before immediately writing them to their intended destination.
If the stream did save any characters, the destination was flushed. If the destination included another character or byte stream, then using flush()
only once was sufficient to flush all of the buffer data in a string of Writer and output streams.
The primary goal of the buffering is to enhance the performance of the I/O
operations.
Purpose of the flush()
Method
When we write data to a stream, the data is not instantaneously written. Instead, it is stored in a buffer. When we must be sure that all of the data from the buffer
has been written, we use the flush()
function.
Since we need to be sure that all of the writes have been finished before we can shut the stream, the flush()
function is called at the closing of the file or buffered writer()
. However, we should use flush()
if it is necessary for any of these writes to be preserved at any point before you terminate the stream()
.
When all of the temporary memory locations have reached capacity, we use the flush()
function. This clears out all data streams, ensures they are entirely processed, and makes room for different data streams in the temporary buffer location.
Let’s have an example to understand it clearly. First, we’ll create a string variable and populate it with some data.
String s = "Hey, Muhammad Zeeshan Here! Let's Code!";
The write()
function is then used to send the string to the stream Writer.
w.write(s);
You can instantly send the data to the stream using the flush()
function.
w.flush();
And last, we end the stream using the close()
function.
close();
Complete Source Code:
import java.io.*;
public class Shanii {
public static void main(String[] args) throws Exception {
String s = "Hey, Muhammad Zeeshan Here! Let's Code!";
Writer w = null;
w = new PrintWriter(System.out);
w.write(s);
w.flush();
System.out.println();
w.close();
}
}
Output:
Hey, Muhammad Zeeshan Here! Let's Code!
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn