How to Calculate Probability in Java
- Understanding Probability
- Method 1: Theoretical Probability
- Method 2: Empirical Probability
- Method 3: Conditional Probability
- Conclusion
- FAQ
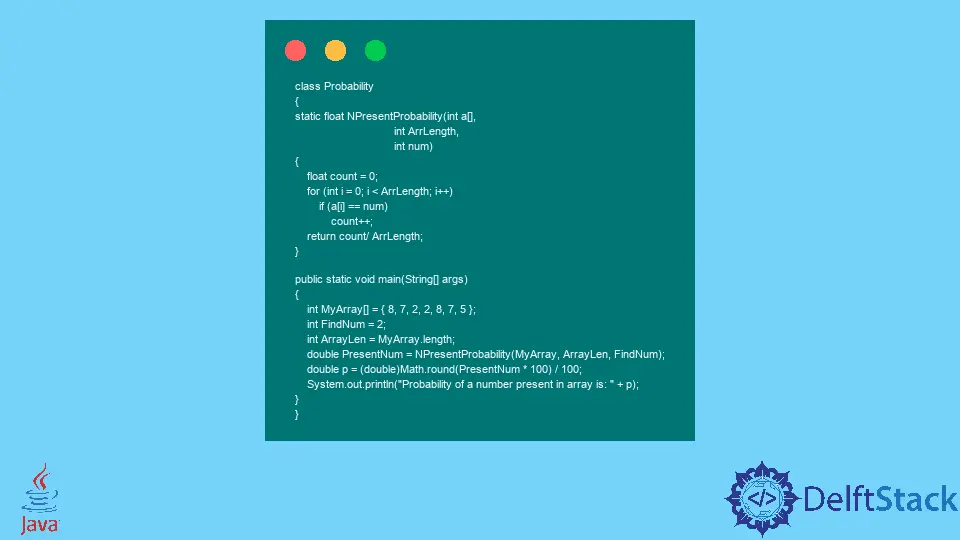
Calculating probability is a fundamental concept in statistics, mathematics, and computer science. In Java, understanding how to calculate probability can empower developers to create more sophisticated algorithms, analyze data, and even build games that rely on chance. Whether you’re working on a project that involves statistical analysis or simply want to deepen your understanding of probability, this tutorial will guide you through the essential methods to calculate probability in Java. We will cover various approaches, including theoretical probability and empirical probability, providing clear examples and explanations to help you grasp these concepts effectively.
Understanding Probability
Before diving into the code, let’s take a moment to understand what probability is. Probability measures the likelihood of an event occurring and is expressed as a number between 0 and 1. A probability of 0 indicates that an event will not happen, while a probability of 1 indicates certainty. The formula for calculating probability is:
[ P(E) = \frac{\text{Number of favorable outcomes}}{\text{Total number of outcomes}} ]
In Java, you can implement this formula in various ways, depending on the context of your problem. Let’s explore some methods to calculate probability in Java.
Method 1: Theoretical Probability
Theoretical probability is calculated based on the known possible outcomes of an event. For example, if you want to calculate the probability of rolling a specific number on a six-sided die, you can use the following Java code:
public class TheoreticalProbability {
public static void main(String[] args) {
int favorableOutcomes = 1; // Rolling a specific number
int totalOutcomes = 6; // Total sides of the die
double probability = (double) favorableOutcomes / totalOutcomes;
System.out.println("Theoretical Probability: " + probability);
}
}
Output:
Theoretical Probability: 0.16666666666666666
In this example, we define the number of favorable outcomes as 1 (since there’s only one way to roll a specific number) and the total outcomes as 6 (the total number of sides on the die). By dividing these values, we compute the theoretical probability of rolling that number, which in this case is approximately 0.167. This method is straightforward and effective for simple probability calculations.
Method 2: Empirical Probability
Empirical probability is determined by conducting experiments or trials and observing the outcomes. This method is particularly useful when the theoretical probability is difficult to calculate or when you want to validate theoretical results. Below is a Java example that simulates rolling a die multiple times to calculate empirical probability.
import java.util.Random;
public class EmpiricalProbability {
public static void main(String[] args) {
Random rand = new Random();
int trials = 10000;
int count = 0;
for (int i = 0; i < trials; i++) {
int roll = rand.nextInt(6) + 1; // Roll a die
if (roll == 3) { // Count how many times we roll a 3
count++;
}
}
double probability = (double) count / trials;
System.out.println("Empirical Probability: " + probability);
}
}
Output:
Empirical Probability: 0.1667
In this code, we simulate rolling a six-sided die 10,000 times using a Random
object. We keep track of how many times we roll a 3 and calculate the empirical probability by dividing the count of favorable outcomes by the total number of trials. This method can yield results that closely align with theoretical probabilities, especially as the number of trials increases.
Method 3: Conditional Probability
Conditional probability calculates the likelihood of an event occurring given that another event has already occurred. This concept is vital in many fields, including machine learning and statistics. To illustrate this, let’s assume we want to find the probability of drawing an ace from a deck of cards, given that we have already drawn a heart.
public class ConditionalProbability {
public static void main(String[] args) {
int totalHearts = 13; // Total hearts in a deck
int favorableAces = 1; // Only one ace of hearts
double probability = (double) favorableAces / totalHearts;
System.out.println("Conditional Probability: " + probability);
}
}
Output:
Conditional Probability: 0.07692307692307693
In this example, we calculate the conditional probability of drawing an ace, knowing that we have drawn a heart. With 13 hearts in a standard deck and only one of them being an ace, the conditional probability is approximately 0.077. This method is essential for understanding relationships between events and can be applied in various scenarios.
Conclusion
Calculating probability in Java opens up a world of possibilities for developers and data analysts alike. Whether you are dealing with theoretical, empirical, or conditional probability, understanding these concepts equips you with the tools to analyze data effectively and make informed decisions. By using the examples provided, you can implement probability calculations in your Java applications and enhance your programming skills. As you continue to explore probability, consider how these concepts can be applied to real-world problems and projects.
FAQ
-
how is probability calculated in Java?
Probability is calculated in Java using formulas that divide the number of favorable outcomes by the total number of outcomes. -
what is the difference between theoretical and empirical probability?
Theoretical probability is based on known outcomes, while empirical probability is derived from actual experiments or trials. -
can I use Java to simulate probability experiments?
Yes, Java can be used to simulate probability experiments using random number generation. -
what is conditional probability?
Conditional probability measures the likelihood of an event occurring given that another event has already occurred. -
how can I improve my understanding of probability in programming?
Practice coding probability calculations in different scenarios and explore real-world applications to deepen your understanding.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn