Private Static Variable in Java
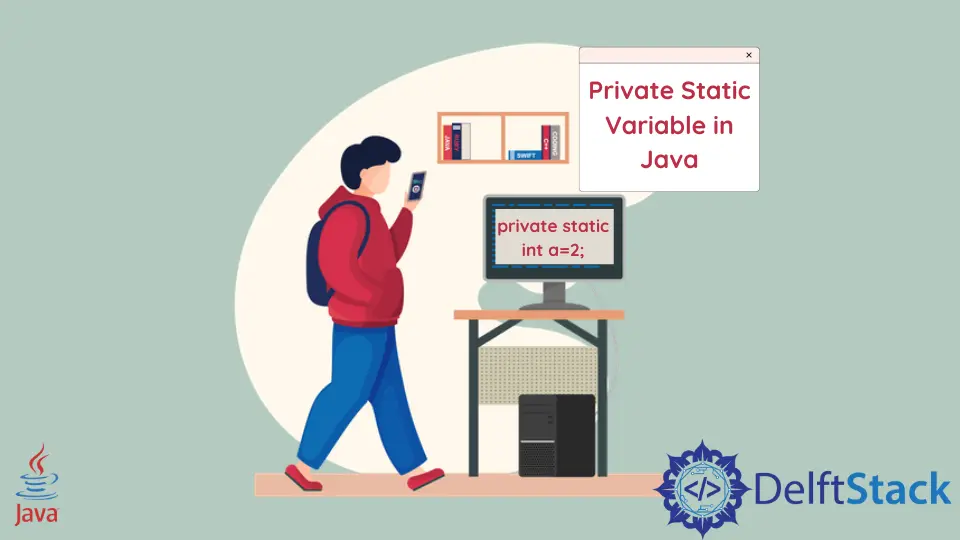
Class variables, commonly known as static variables, are defined using the static
keyword in a class but outside a method, constructor (default or parameterized), or block.
Private static variables are frequently utilized for constants. For example, many individuals prefer not to use constants in their code. Instead, they prefer to create a private static variable with a meaningful name and utilize it in their code, making the code more understandable.
If a variable is declared static, then the variable’s value is the same for all the instances, and we don’t need to create an object to call that variable.
A variable declared private static could easily be accessed, but only from the inside of the class in which it is defined and declared. It is because the variable is declared private, and private variables are not accessible outside the class. Within the class, they can be accessed using ClassName.Variable_name
.
For example,
public class Demo {
private static int a = 10;
public static void main(String[] args) {
System.out.println(Demo.a); // className.variable_name
}
}
Output:
10
In the above example, we created a static private variable and printed its value.
Let us understand an example to see the difference between a private and a private static variable.
public class test {
private static int eye = 2;
private int leg = 3;
public test(int eyes, int legs) {
eye = eyes;
leg = leg;
}
public test() {}
public void print() {
System.out.println(eye);
System.out.println(leg);
}
public static void main(String[] args) {
test PersonB = new test(14, 8);
PersonB.print();
}
}
Output:
14
3
The PersonB
object changes the eye
variable in the above example, but the leg
variable remains the same. This is because a private variable copies itself to the method, preserving its original value. But a private static value only has one copy for all methods to share, thus changing its value changes the original value.