Pi Constant in Java
- Using Math.PI for Simple Calculations
- Calculating Area of a Circle
- Implementing Pi in Other Geometric Calculations
- Using Pi in Trigonometric Functions
- Conclusion
- FAQ
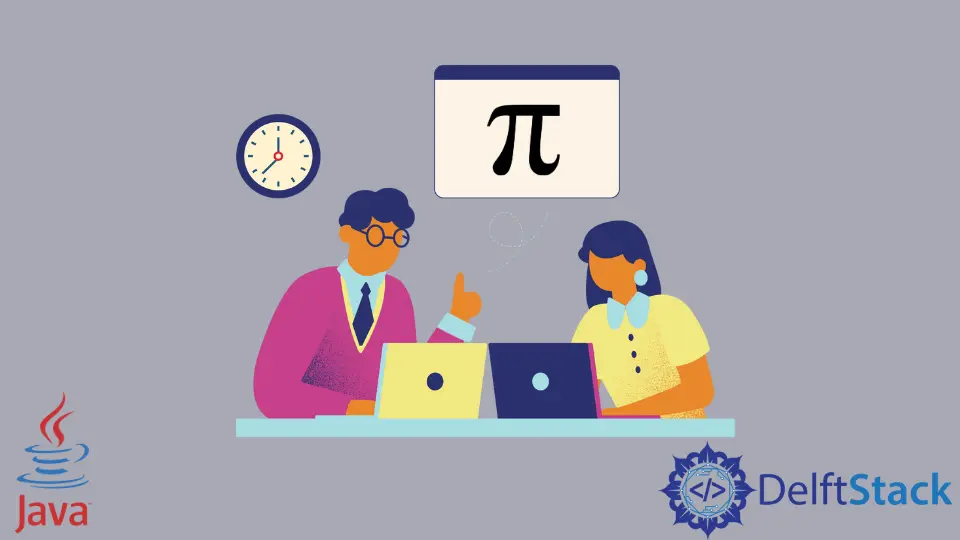
Understanding the value of pi is crucial for anyone delving into mathematics or programming. In Java, the pi constant is readily available, making calculations involving circles, spheres, and other geometric shapes a breeze.
This tutorial will walk you through the various ways to use the pi constant in Java, from simple calculations to more complex applications. Whether you’re a beginner or an experienced developer, you’ll find practical examples and clear explanations that will enhance your understanding of how to implement pi in your Java projects. Let’s dive in and explore the fascinating world of pi in Java!
Using Math.PI for Simple Calculations
One of the simplest and most effective ways to access the value of pi in Java is through the Math
class. Java’s Math.PI
provides a double value of pi, which is approximately 3.14159. This built-in constant allows you to perform various mathematical operations easily.
Here’s a basic example to calculate the circumference of a circle:
public class Circle {
public static void main(String[] args) {
double radius = 5.0;
double circumference = 2 * Math.PI * radius;
System.out.println("Circumference: " + circumference);
}
}
Output:
Circumference: 31.41592653589793
In this example, we define a radius for our circle and use the formula for circumference (C = 2 * π * r) to calculate it. The Math.PI
constant ensures that we have a precise value of pi for our calculations. This method is not only straightforward but also efficient, as it leverages Java’s built-in functionality, allowing you to focus on more complex programming tasks.
Calculating Area of a Circle
Building upon the previous example, let’s explore how to calculate the area of a circle using the pi constant in Java. The area can be computed using the formula A = π * r². Here’s how you can implement this in code:
public class CircleArea {
public static void main(String[] args) {
double radius = 5.0;
double area = Math.PI * Math.pow(radius, 2);
System.out.println("Area: " + area);
}
}
Output:
Area: 78.53981633974483
In this code snippet, we again define a radius. This time, we use the formula for the area of a circle, which involves squaring the radius. The Math.pow
method is utilized to raise the radius to the power of 2. By using Math.PI
, we ensure that our area calculation is accurate and reliable. This approach is particularly useful for applications involving geometry, physics, or any field where circular calculations are essential.
Implementing Pi in Other Geometric Calculations
Beyond circles, pi is also vital in calculations involving spheres and cylinders. For instance, if you want to compute the volume of a sphere, you can use the formula V = (4/3) * π * r³. Here’s how to implement this in Java:
public class SphereVolume {
public static void main(String[] args) {
double radius = 5.0;
double volume = (4.0 / 3.0) * Math.PI * Math.pow(radius, 3);
System.out.println("Volume: " + volume);
}
}
Output:
Volume: 523.5987755982989
In this example, we calculate the volume of a sphere using the appropriate formula. The use of Math.PI
again ensures that we have the most accurate value of pi. This method is especially useful for simulations or applications that require volumetric calculations, such as in engineering or 3D modeling.
Using Pi in Trigonometric Functions
Pi plays a significant role in trigonometry, especially when working with angles. In Java, the Math
class provides trigonometric functions such as sin
, cos
, and tan
, which expect angles in radians. Since π radians equal 180 degrees, you can use the pi constant to convert degrees to radians. Here’s an example demonstrating this:
public class Trigonometry {
public static void main(String[] args) {
double angleDegrees = 90.0;
double angleRadians = Math.toRadians(angleDegrees);
double sineValue = Math.sin(angleRadians);
System.out.println("Sine of 90 degrees: " + sineValue);
}
}
Output:
Sine of 90 degrees: 1.0
In this code, we convert an angle from degrees to radians using Math.toRadians
. Then, we calculate the sine of that angle using Math.sin
. This is particularly useful in applications involving oscillations, waves, or any scenario where trigonometric calculations are necessary.
Conclusion
In this tutorial, we explored the various ways to utilize the pi constant in Java. From simple calculations involving circles to more complex geometric shapes and trigonometric functions, Math.PI
provides a reliable and accurate representation of pi. By leveraging Java’s built-in functionalities, you can streamline your coding process and focus on developing robust applications. Whether you’re building a simple program or a complex system, understanding how to work with pi will undoubtedly enhance your programming toolkit.
FAQ
-
What is the value of pi in Java?
The value of pi in Java can be accessed usingMath.PI
, which is approximately 3.14159. -
Can I use pi for calculations other than circles?
Yes, pi is used in various calculations, including spheres, cylinders, and trigonometric functions. -
How do I convert degrees to radians in Java?
You can convert degrees to radians in Java by using theMath.toRadians(degrees)
method. -
Is there a way to define my own pi constant in Java?
While you can define your own constant, it is recommended to useMath.PI
for accuracy. -
What are some practical applications of pi in programming?
Pi is commonly used in fields such as physics, engineering, computer graphics, and any application involving circular or oscillatory motion.