How to Pass by Reference in Java
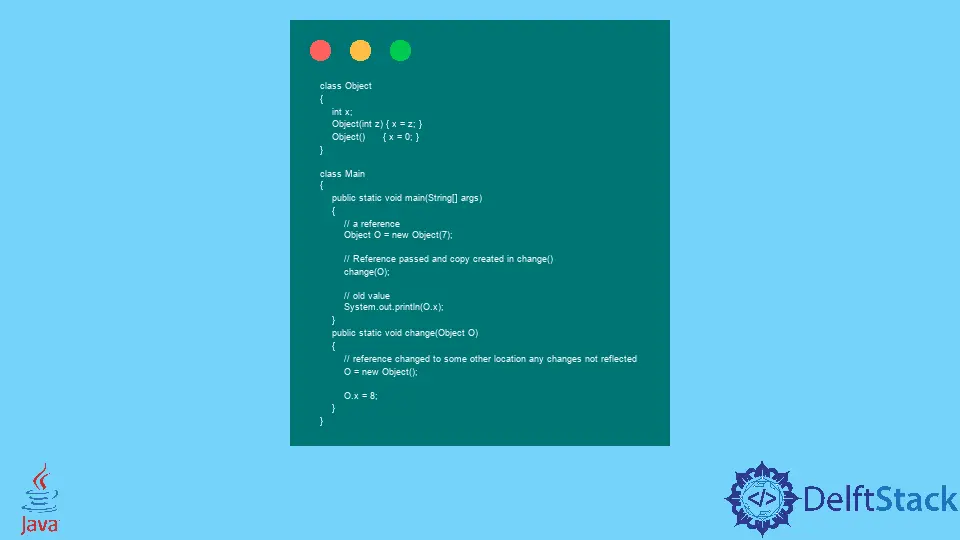
A pointer is a variable in programming language architecture that implicitly monitors the address of specific data. An equivalent for another variable is called a reference. Any modifications made to the reference variable have a direct impact on the original.
Java is a pass-by-value language. It states so itself in its specifications it was never a pass-by-reference language. In Java, a lot of confusion exists between the two terms due to the ambiguous nature of references. We will try to clear it up in this article.
Let us discuss pass-by-value. Here, the value of a formal parameter is copied into a location used to store it during method/function execution. This location typically has a chunk of memory allocated for that purpose.
In pass-by-reference, the value of a formal parameter is copied into a location used to store it during method/function execution. This location typically has a chunk of memory allocated for that purpose.
Java is promoted as a robust and secure language, and one of Java’s benefits is that it didn’t enable pointer like C++. They even chose a different term for the notion, officially referring to them as “references”.
The key to grasping this is to consider something like
Object Obj;
It’s a pointer to the Object
, not an Obj
. The term reference is misunderstood in Java and is the source of most of the confusion. In other programming languages, what they call references to work as pointers.
When we talk about objects in Java, we’re talking about object-handles called references, which are also supplied by value.
In the above code, you’re effectively sending the constructed Object
address to the method.
Java is similar to C in that it is a procedural language. You can assign a pointer to a method, send it there, trace it inside the method, and change the data it pointed to. Any changes you make to the pointer’s position, on the other hand, will go unnoticed by the caller. (In a pass-by-reference language, the method function can change the pointer without the caller realizing.)
Consider reference parameters to be substituted for passed-in variables. The variable that we passed in is assigned when that alias is assigned.
Let us understand this better with two situations.
If we alter the object itself and point it to another place or object, the changes are not reflected. The changes won’t be reflected in the main()
function if we have given the reference to a different place.
See the code below.
class Object {
int x;
Object(int z) {
x = z;
}
Object() {
x = 0;
}
}
class Main {
public static void main(String[] args) {
// a reference
Object O = new Object(7);
// Reference passed and copy created in change()
change(O);
// old value
System.out.println(O.x);
}
public static void change(Object O) {
// reference changed to some other location any changes not reflected
O = new Object();
O.x = 8;
}
}
Output:
7
Now, Suppose we don’t assign a reference to a new place or object. In that case, the results are mirrored back: You may make modifications to the members. These changes are reflected if we do not alter the reference to refer to another object (or memory location).
For example,
class Object {
int x;
Object(int z) {
x = z;
}
Object() {
x = 0;
}
}
class Main {
public static void main(String[] args) {
// a reference
Object O = new Object(7);
// Reference passed and copy created in change()
change(O);
// old value
System.out.println(O.x);
}
public static void change(Object O) {
O.x = 8;
}
}
Output:
8