Parameters vs Arguments in Java
- What are Parameters in Java
- What are Arguments in Java
- Differences between Arguments and Parameters in Java
- Conclusion
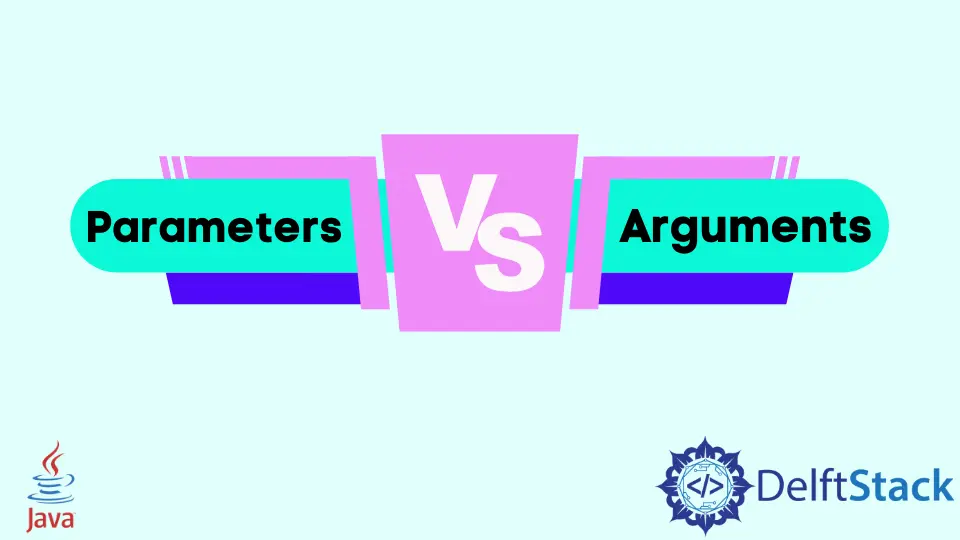
This tutorial introduces the difference between parameters and arguments with examples in Java.
Parameters and arguments are the most used terms in computer programming. Whenever we write a program, there is a high probability of using a function/method. The concept of the method has two terms associated with it, the first is the arguments, and the second is parameters.
In this tutorial, we will discuss arguments and parameters in detail.
What are Parameters in Java
Parameters are the variables that are present in the method definition. We use these variables inside the method for data manipulations.
The parameters have local scope as they can only be used in the method. These variables make the method’s execution easier. Let’s see an example to understand what we’re talking about:
public int multiply(int par1, int par2) {
int result = par1 * par2;
return result;
}
In the example code above, par1
and par2
are the parameters of the method multiply()
. These are two local variables having a function-specific lifespan. They can also accept any values passed to the method when it’s called.
What are Arguments in Java
The variables provided to the method during calling for execution are called arguments.
Furthermore, the method’s local variables take the values of the arguments and can thus process these parameters for the final output. The arguments are the real values we provide as input to obtain the desired result.
Let’s see an example to understand what we’re talking about:
public static void main(String args[]) {
int arg1 = 90;
int arg2 = 50;
int r = multiply(arg1, arg2); // arg1 and arg2 are the arguments for this method
}
In the example code above, arg1
and arg2
are the arguments. Since arguments are real values, in our example, 90
and 50
are used as arguments values.
Let’s understand with a complete example.
public class SimpleTesting {
public static void main(String args[]) {
int arg1 = 90;
int arg2 = 50;
int r = multiply(arg1, arg2); // arg1 and arg2 are the arguments
System.out.println("result = " + r);
}
public static int multiply(int par1, int par2) {
int result = par1 * par2;
return result;
}
}
Output:
result = 4500
Differences between Arguments and Parameters in Java
In this section, we will tackle the differences between arguments and parameters. To better understand, look at the table below. We provide a summarized comparison here.
Arguments | Parameters |
---|---|
It is used to send values from the calling method to the receiving method | They are defined when the function is defined |
It is also known as actual parameter or actual argument | It is also known as a formal parameter or formal argument |
An argument is a nameless expression that can be a variable, a constant, or a literal. | A parameter has a name, a data type, and a method of being called (call by reference or call by value) |
Important points
- The total number of parameters in a method definition should match the number of arguments in a method call. The method with variable-length parameter lists is an exception to this rule.
- In a method call, the data type of arguments should match the data type of parameters in the method specification.
Conclusion
In this article, we learned what arguments and parameters are. Argument and parameter are frequently used interchangeably. We now know what both of these terms exactly mean in Java programming.