Annotation @param in Java
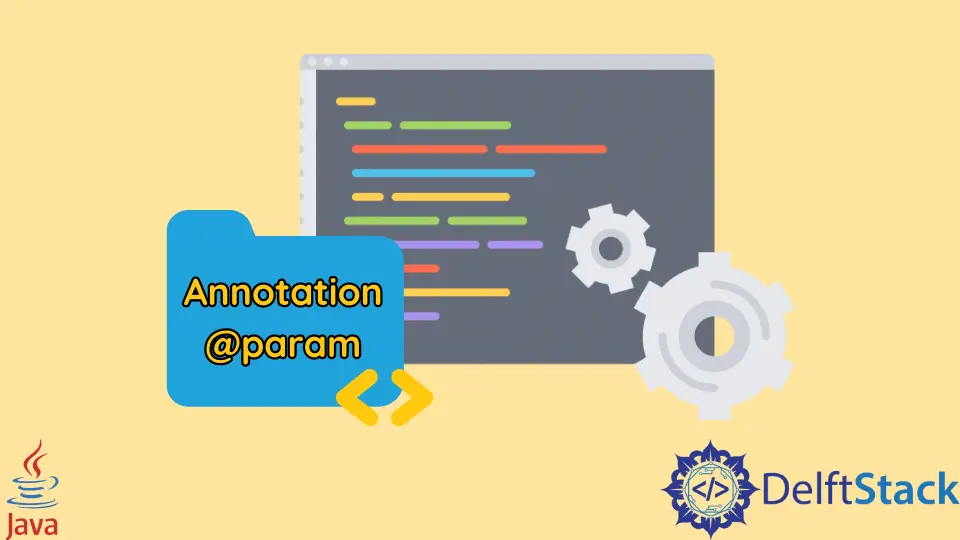
In Java, annotations are the tags that represent the metadata, which is attached with a class, interface, methods, etc., to indicate some special type of additional information that can be used by JVM and Java compiler.
The @param
annotation is a special format comment used by the javadoc
that generates documentation.
In this tutorial, we will discuss how does the annotation @param
works in Java.
As mentioned above, @param
is a special type of format comment used by the javadoc
, that generates the documentation. It denotes a description of the parameter (or multiple parameters) a method may receive.
There are also annotations like @return
and @see
to describe the return values and related information.
If used in any method specifically, this annotation will not affect the method of its working in any way. It is just used for creating documentation of that particular method. We can put this annotation right before a class, method, field, constructor, interface, etc.
The advantage to using this annotation is that by using this, we allow the simple Java classes, which might be containing attributes and some custom javadoc
tags, to serve as simple metadata descriptions for the code generation.
For example,
/*
*@param number
*@return integer
*/
public int main number(int num) {
// if number is less than 5, square it
if (num < 5) {
num = num * num;
} else {
// else add the number to itself
num = num + num;
}
return num;
}
In the above example, method number()
will act as the metadata for the rest of the code. Whenever the code reuses this method, IDE shows up the parameters this method accepts. In this case, one parameter is accepted, and that is an integer named num
. Also, the return type of method, which in this case is int
.