How to Override equals() in Java
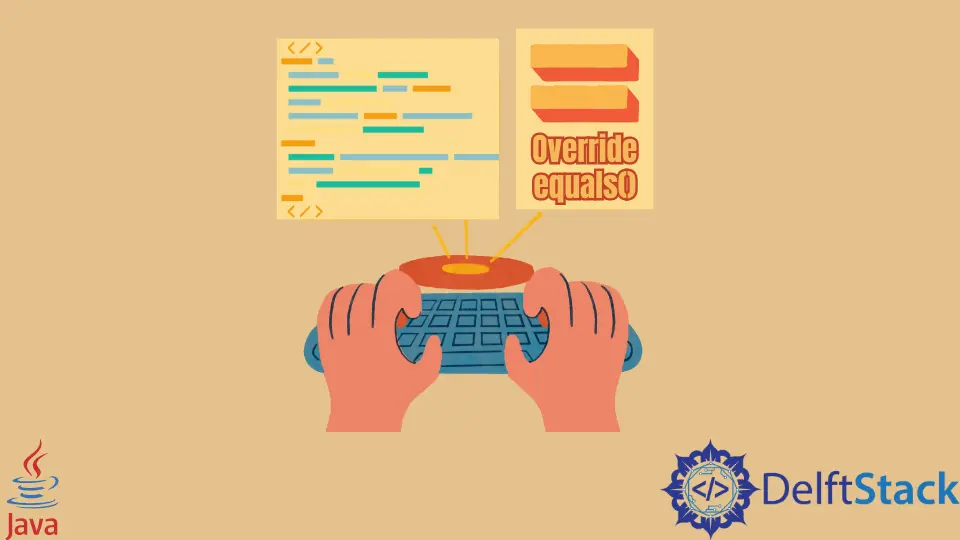
In Java, Overriding is when the child class or the subclass has the same execution of method as declared in the parent class.
The equals()
method compares two strings. If the data of one string object is the same as the other, it returns True value otherwise False. When we override the equals()
method, it is always recommended to override the hashtag()
method also.
This tutorial will demonstrate how to override equals in Java.
To check whether the values in the objects are equal or not, we use the equals()
method. We can override this method in the class to check whether the two objects have the same data or not, as the classes in Java are inherited from the object classes only.
The @Override
tells the compiler of overriding during compile time.
See the code given below:
class Complex {
private double ab, cd;
public Complex(double ab, double cd) {
this.ab = ab;
this.cd = cd;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (!(o instanceof Complex)) {
return false;
}
Complex c = (Complex) o;
return Double.compare(ab, c.ab) == 0 && Double.compare(cd, c.cd) == 0;
}
}
public class Main {
public static void main(String[] args) {
Complex c1 = new Complex(12, 13);
Complex c2 = new Complex(12, 13);
if (c1.equals(c2)) {
System.out.println("Equal ");
} else {
System.out.println("Not Equal ");
}
}
}
Output:
Equal
In the above example, overriding is done by the equals()
method to compare two complex objects with the help of Boolean expression. Then, if the object is compared with itself, it returns True. Then, we have checked whether the object is an instance of complex or not and hence returns False. Now, we typecast the object into complex so that we can compare the data members. Finally, we make driver class to test the complex class.