How to Fix Error: Not on FX Application Thread in Java
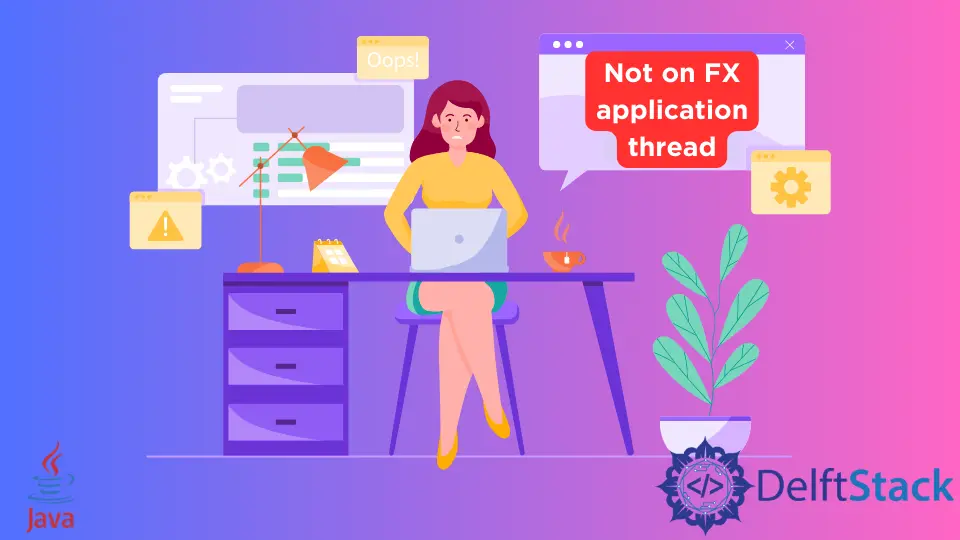
This tutorial demonstrates the Not on FX application thread
error in Java.
the Not on FX application thread
in Java
The error Not on FX application thread
occurs when we try to call a method from a thread that is not an FX thread and should be called from the FX thread. This was not a problem with previous versions of JavaFX.
It occurs with the new implementation of JavaFX 8. This error occurs when changing the user interface in different threads in the JavaFX application.
Here is an example that will throw the Not on FX application thread
error.
Thread DemoThreadShow = new Thread(new Runnable() {
@Override
public void run() {
try {
newthread.setStyle("visibility: true");
Thread.sleep(10000);
Thread.interrupted();
} catch (Exception e) {
thread.setText("" + Integer.valueOf(thread.getText()) + 5);
newthread.setStyle("visibility: false");
}
}
});
DemoThreadShow.start();
The code above creates a thread and applies some methods to that thread. These methods are from the JavaFX application.
When applying the setText
method, it will throw the error Exception in thread "Thread-5" java.lang.IllegalStateException: Not on FX application thread; currentThread = Thread-5
.
The following changes can be made to the code to solve this issue.
-
To make UI changes while working on FX applications and threads, use the following method.
Platform.runLater(() -> { // GUI STUFF }); or : Platform.runLater(new Runnable() { @Override public void run() { // GUI STUFF } });
-
We can also use the
Service
andTask
rather than theThread
.Service
has many more features thanThread
.Changing the UI inside the task will never throw the
Not on FX application thread
error. Here is a code example of usingService
andTask
for this purpose.Service New_Service = new Service() { @Override protected Task createTask() { return new Task() { @Override protected Object call() throws Exception { Platform.runLater(() -> { // GUI stuff here }); return null; } }; } }; New_Service.start();
The code above is a more convenient way than threads.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn FacebookRelated Article - Java Thread
- Overview of ThreadLocal in Java
- Daemon Thread in Java
- How to Get Thread Id in Java
- How to Kill Thread in Java
Related Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack