Not Equals in Java
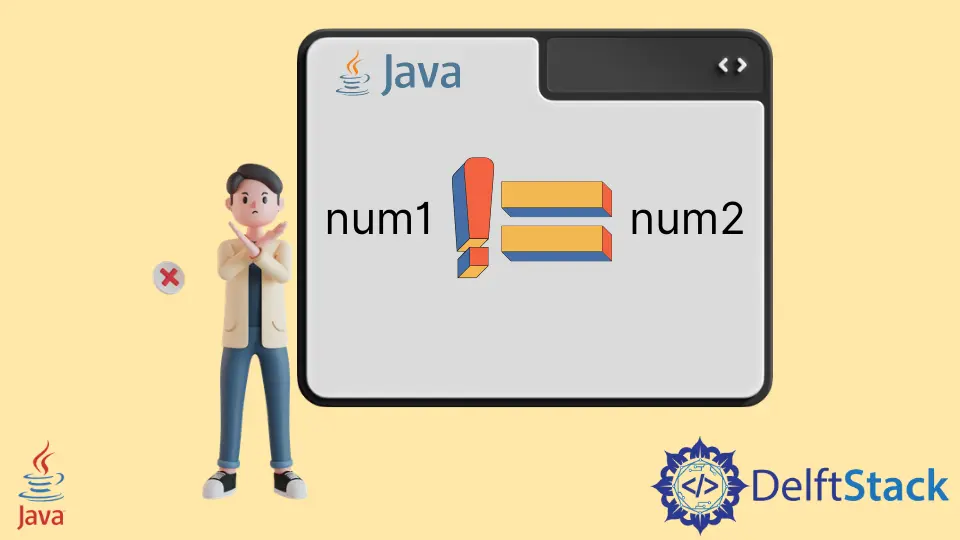
This article shows how to use the !=
operator that we also call the not equals operator. We can also use !
with the equals()
method to check the non-equality of the data.
Using the Not Equals Operator in Java
The most basic way to use the not equals operator is to check for equality between two variables.
The program has two int
variables, num1
and num2
. Here, num1
contains the value 123
, and the num2
variable has 321
.
We create an if
condition to check if the variables match or not. In the condition, write num1 != num2
where the variable on the left side of the operator is compared. The variable is on the right side of the operator.
The true
block of the if
condition executes when the condition is not met (when num1
is not equal to num2
), and if they match, then the false
block is executed.
As both the variables have different values, the true
block of the condition executes.
Sample Code:
public class JavaExample {
public static void main(String[] args) {
int num1 = 123;
int num2 = 321;
if (num1 != num2) {
System.out.println("str1 and str2 are not equal");
} else {
System.out.println("str1 and str2 are equal");
}
}
}
Output:
str1 and str2 are not equal
Using the Not Equals Operator With equals()
We can use the !
operator with the equals()
method to check if the contents of the variables match or not.
In the example, we take two String variables. In the if
condition, we check the str1.equals(str2)
with a !
operator at the beginning.
The !
operator makes the result opposite, which means if the str1.equals(str2)
statement returns true
as a result, the operator !
makes it false.
So, in our cases, we check if the str1.equals(str2)
throws true
, and if yes, we use the operator, which proves that the variables are not the same.
public class JavaExample {
public static void main(String[] args) {
String str1 = "String A";
String str2 = "String B";
if (!str1.equals(str2)) {
System.out.println("str1 and str2 are not equal");
} else {
System.out.println("str1 and str2 are equal");
}
}
}
Output:
str1 and str2 are not equal
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn