Multiple Action Listeners in Java
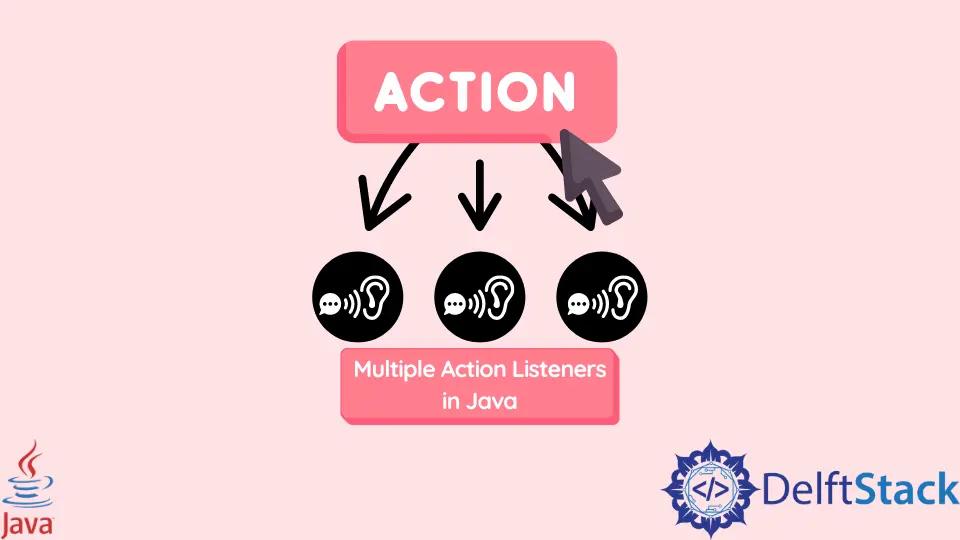
This article will teach us how to create multiple action listeners in Java.
In Java, ActionListener
is a class for handling the action events. So Java provides this interface using which we can find where the user clicked the button and generate some event to perform an action like print something or calculate something whenever the button is clicked.
So, here we’ll see how to create multiple action listeners for multiple buttons in our Java Swing project.
First, let’s see how to write an action listener.
Write an ActionListener
in Java
In Java, java.awt.event
package contains the ActionListener
interface which contains only actionPerformed()
in it. As it is an interface, the common way to write the ActionListener
is by implementing the ActionListener
interface.
We have to follow these steps when implementing the ActionListener
.
-
Our class should implement the
ActionListener
interface.public class test implements ActionListener
-
Add the component like a button with the listener.
Button b = new Button("Click Here"); b.addActionListener(this);
-
The last step is to override the only interface method, i.e.
actionPerformed()
method.
Example code:
import java.awt.*;
import java.awt.event.*;
public class test implements ActionListener // 1st step
{
public test() // constructor
{
Button b = new Button("Click Here");
// 2nd step
b.addActionListener(this);
// our code.....
}
// 3rd step
public void actionPerformed(ActionEvent evt) {
// our code
}
public static void main(String args[]) {
Test t = new Test();
}
}
Sometimes anonymous classes are also used to implement the ActionListener
; it is the most preferred way as it is an easy and concise way of writing.
Button b = new Button("Click Here");
b.addActionListner(new ActionListener() {
@Override
public void actionPerformed(ActionEvent evt) {
// some action
}
});
Multiple Action Listeners in Java
We can create multiple action listeners just by extending the things discussed above.
Example code: Using anonymous classes
JButton multiplyButton = new JButton(new AbstractAction("multiply") {
@Override
public void actionPerformed(ActionEvent evt) {
// some action
}
});
JButton divideButton = new JButton(new AbstractAction("divide") {
@Override
public void actionPerformed(ActionEvent evt) {
// some action
}
});