How to Merge PDF in Java
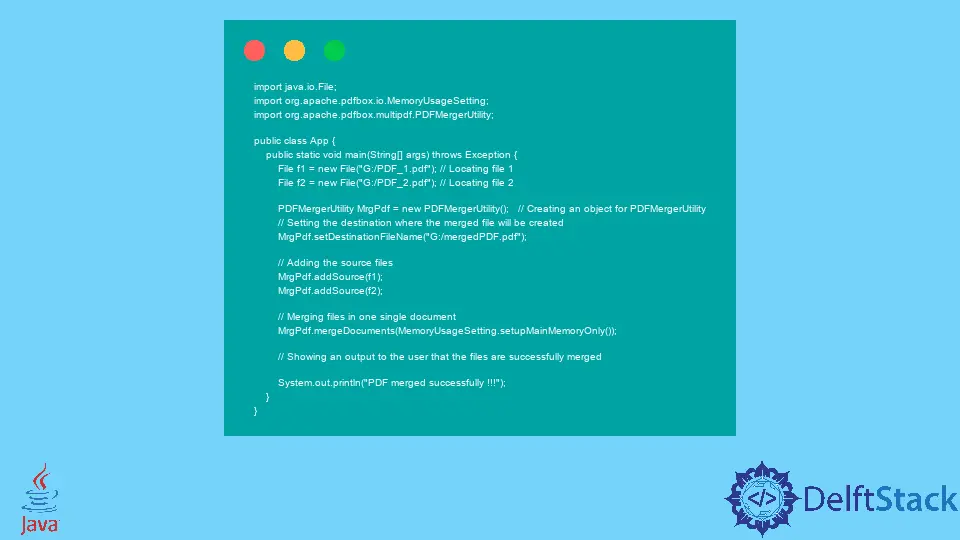
Sometimes we need to combine multiple PDF files and merge them into one PDF file. We can easily do this task using the most popular Apache library, PDFBox
in Java.
This article will show how we can merge multiple PDF files in Java and the necessary example and explanations to clarify the topic.
Use the PDFBox
to Merge PDF in Java
In our example below, we will illustrate how we can merge two different PDFs using the PDFBox
.
Suppose we have two PDF files with the below contents.
PDF_1.pdf
This is line 1 of pdf 1
This is line 2 of pdf 1
This is line 3 of pdf 1
This is line 4 of pdf 1
PDF_2.pdf
This is line 1 of pdf 2
This is line 2 of pdf 2
This is line 3 of pdf 2
This is line 4 of pdf 2
Now the code for the example that will merge both of these PDF files will be as follows:
import java.io.File;
import org.apache.pdfbox.io.MemoryUsageSetting;
import org.apache.pdfbox.multipdf.PDFMergerUtility;
public class App {
public static void main(String[] args) throws Exception {
File f1 = new File("G:/PDF_1.pdf"); // Locating file 1
File f2 = new File("G:/PDF_2.pdf"); // Locating file 2
PDFMergerUtility MrgPdf = new PDFMergerUtility(); // Creating an object for PDFMergerUtility
// Setting the destination where the merged file will be created
MrgPdf.setDestinationFileName("G:/mergedPDF.pdf");
// Adding the source files
MrgPdf.addSource(f1);
MrgPdf.addSource(f2);
// Merging files in one single document
MrgPdf.mergeDocuments(MemoryUsageSetting.setupMainMemoryOnly());
// Showing an output to the user that the files are successfully merged
System.out.println("PDF merged successfully !!!");
}
}
The purpose of each line is left as a comment. After executing the above example, you will get an output like the one below.
PDF merged successfully !!!
And you will see that a merged PDF file is created in the directory we provided with the contents below.
Page 1:
This is line 1 of pdf 1
This is line 2 of pdf 1
This is line 3 of pdf 1
This is line 4 of pdf 1
Page 2:
This is line 1 of pdf 2
This is line 2 of pdf 2
This is line 3 of pdf 2
This is line 4 of pdf 2
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn