The Max Value of an Integer in Java
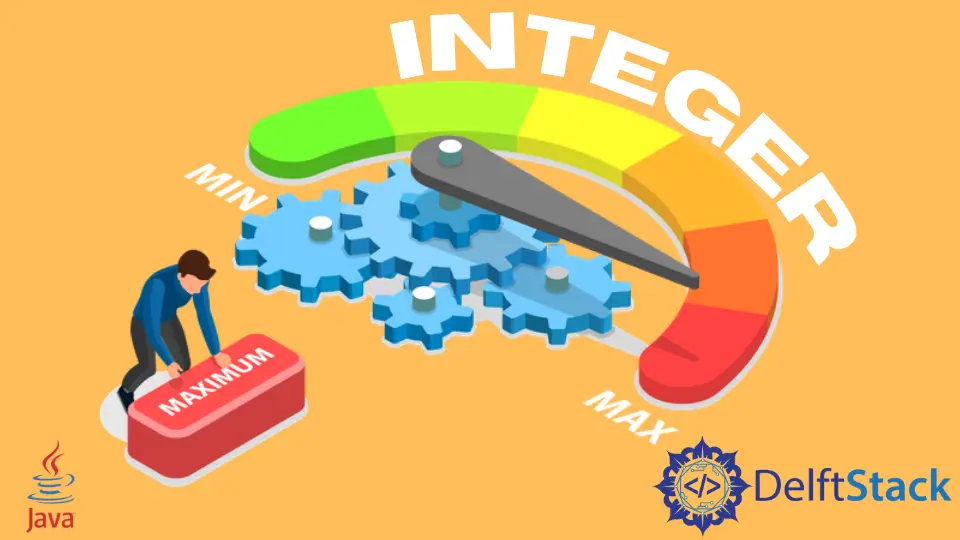
Java is a versatile programming language that is widely used for building robust applications. One common task that developers often encounter is determining the maximum value of an integer in Java. Understanding how to retrieve this value is crucial, especially when working with numerical data, as it helps prevent overflow errors and ensures that calculations remain accurate.
In this article, we will explore different methods to obtain the maximum value of an integer in Java, providing clear examples and explanations along the way. Whether you’re a beginner or an experienced programmer, this guide will equip you with the knowledge you need to handle integer values effectively.
Using Integer.MAX_VALUE
One of the simplest ways to find the maximum value of an integer in Java is by utilizing the built-in constant Integer.MAX_VALUE
. This constant represents the maximum value that an integer can hold, which is 2,147,483,647. Here’s how you can access and print this value:
public class MaxIntegerValue {
public static void main(String[] args) {
int maxIntValue = Integer.MAX_VALUE;
System.out.println("The maximum value of an integer in Java is: " + maxIntValue);
}
}
Output:
The maximum value of an integer in Java is: 2147483647
In the code above, we first declare a variable maxIntValue
and assign it the value of Integer.MAX_VALUE
. This constant is predefined in the Java programming language, making it easy to access without needing any complex calculations. Next, we print the value to the console. This method is straightforward and efficient, especially for those who are new to Java programming. By using Integer.MAX_VALUE
, you not only save time but also reduce the risk of errors that may occur when manually calculating the maximum integer value.
Using Custom Calculation
While using Integer.MAX_VALUE
is the most common approach, you might want to calculate the maximum integer value programmatically for educational purposes or to understand how it works under the hood. Here’s how you can do that:
public class MaxIntegerValueCalc {
public static void main(String[] args) {
int maxIntValue = 0;
while (maxIntValue >= 0) {
maxIntValue++;
}
System.out.println("The maximum value of an integer calculated is: " + (maxIntValue - 1));
}
}
Output:
The maximum value of an integer calculated is: 2147483647
In this example, we initialize maxIntValue
to zero and use a while
loop to increment it until it becomes negative. When the loop exits, maxIntValue
will be one more than the maximum integer value, so we subtract one before printing it. This method demonstrates how integers behave in Java and can help deepen your understanding of numerical limits. However, keep in mind that this approach is less efficient than directly using Integer.MAX_VALUE
, especially since it involves multiple iterations.
Using Bit Manipulation
Another interesting method to determine the maximum value of an integer in Java is through bit manipulation. This technique involves understanding how integers are represented in binary form. Here’s an example:
public class MaxIntegerValueBit {
public static void main(String[] args) {
int maxIntValue = (1 << 31) - 1;
System.out.println("The maximum value of an integer using bit manipulation is: " + maxIntValue);
}
}
Output:
The maximum value of an integer using bit manipulation is: 2147483647
In this code snippet, we use the left shift operator (<<
) to shift the number 1 to the left by 31 bits. This effectively creates the binary representation of the maximum positive integer. By subtracting one, we convert it from the binary representation of the next integer (which would be out of bounds) back to the maximum valid integer. This method is efficient and shows a different way to approach the problem, appealing to those who enjoy delving into the intricacies of binary operations.
Conclusion
In this article, we explored various methods to find the maximum value of an integer in Java. From using the built-in constant Integer.MAX_VALUE
to calculating it programmatically and employing bit manipulation, each approach has its own merits. For most practical purposes, using Integer.MAX_VALUE
is the most efficient and straightforward method. However, understanding the underlying principles behind these calculations can enhance your programming skills and help you write more robust code. Whether you are a beginner or an experienced developer, mastering these techniques will undoubtedly add value to your Java programming toolkit.
FAQ
-
What is the maximum value of an integer in Java?
The maximum value of an integer in Java is 2,147,483,647, which can be accessed usingInteger.MAX_VALUE
. -
Can I exceed the maximum integer value in Java?
Yes, if you attempt to exceed the maximum integer value, it will result in an overflow, causing the value to wrap around to the negative side. -
What happens if I try to store a number larger than
Integer.MAX_VALUE
?
Storing a number larger thanInteger.MAX_VALUE
will lead to an overflow, and the value will not be stored correctly, resulting in unexpected behavior. -
Is there a maximum value for other data types in Java?
Yes, other data types likelong
,short
, andbyte
also have maximum values, which can be found usingLong.MAX_VALUE
,Short.MAX_VALUE
, andByte.MAX_VALUE
, respectively.
- Why is it important to know the maximum value of an integer?
Knowing the maximum value of an integer is important to prevent overflow errors and ensure that calculations remain accurate, especially in applications dealing with large numbers.