Matrix Multiplication in Java
Shubham Vora
Oct 12, 2023
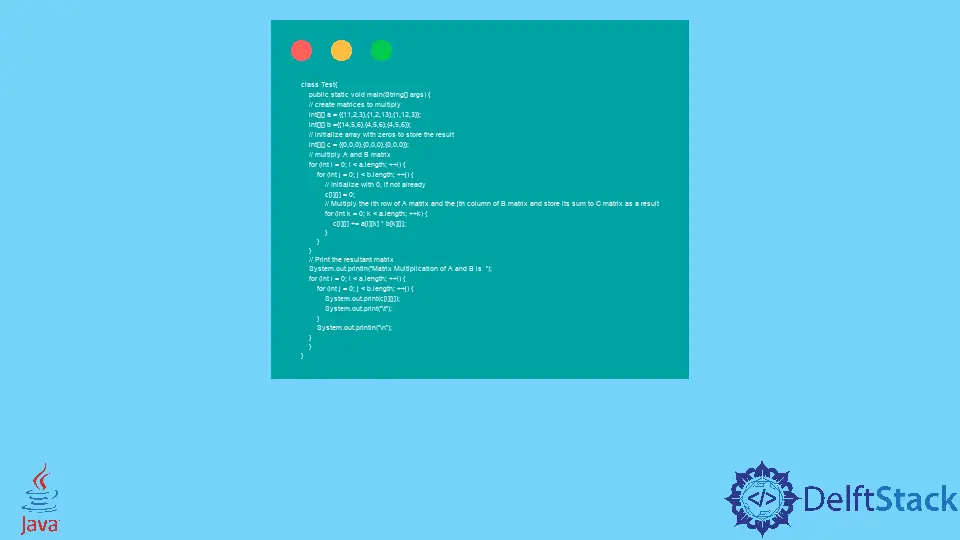
In this article, we will learn to multiply the two matrices in Java.
Multiply Two Matrices In Java
We are using the multiplication and addition operators to multiply two matrices. Users should follow the below steps to multiply two matrices.
-
Create two matrices and initialize them with values.
-
Create a matrix to store output and initialize it with zeros.
-
Use the nested loop to multiply each row of the 1st matrix with each column of the 2nd matrix. Store the sum of it in the output matrix.
Example Code:
class Test {
public static void main(String[] args) {
// create matrices to multiply
int[][] a = {{11, 2, 3}, {1, 2, 13}, {1, 12, 3}};
int[][] b = {{14, 5, 6}, {4, 5, 6}, {4, 5, 6}};
// initialize array with zeros to store the result
int[][] c = {{0, 0, 0}, {0, 0, 0}, {0, 0, 0}};
// multiply A and B matrix
for (int i = 0; i < a.length; ++i) {
for (int j = 0; j < b.length; ++j) {
// Initialize with 0, if not already
c[i][j] = 0;
// Multiply the ith row of A matrix and the jth column of B matrix and store its sum to C
// matrix as a result
for (int k = 0; k < a.length; ++k) {
c[i][j] += a[i][k] * b[k][j];
}
}
}
// Print the resultant matrix
System.out.println("Matrix Multiplication of A and B is ");
for (int i = 0; i < a.length; ++i) {
for (int j = 0; j < b.length; ++j) {
System.out.print(c[i][j]);
System.out.print("\t");
}
System.out.println("\n");
}
}
}
Output:
Matrix Multiplication of A and B is
174 80 96
74 80 96
74 80 96
Time Complexity of the Matrix Multiplication
The time complexity for the matrix multiplication is O(M^3)
, as we are using 3 nested loops.
Space Complexity of the Matrix Multiplication
The space complexity for the matrix multiplication algorithm is O(M^2)
.
Author: Shubham Vora