How to Wrap Text in JavaFX TextArea
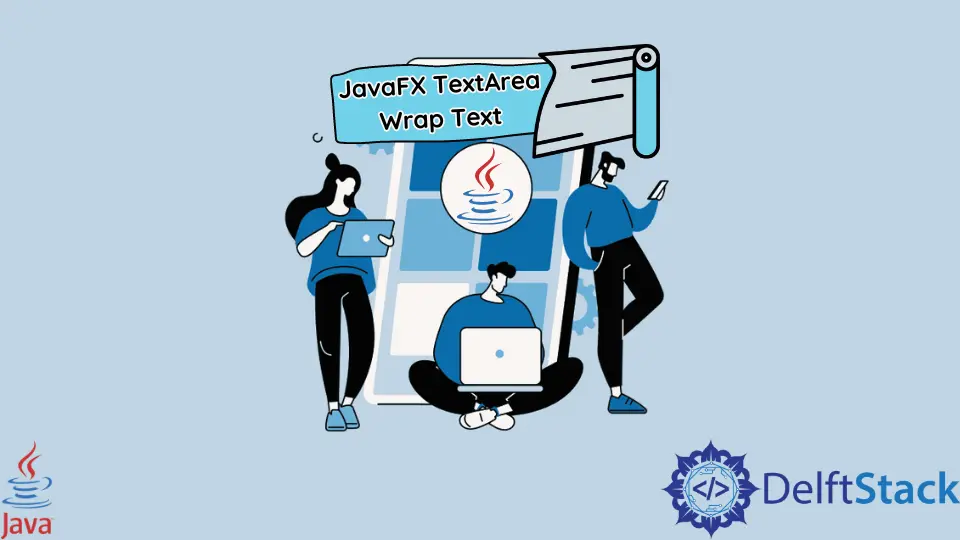
Text areas are used to input the large text. This tutorial demonstrates how to wrap text in TextArea
using JavaFX.
JavaFX TextArea
Wrap Text
The text areas are used to get the large text as input. Sometimes we need to edit some large text and don’t want to write the whole text again; for this purpose, we can wrap the previous text in the text area and edit the part.
The method setWrapText(true)
wraps the text in TextArea
or any other element. Follow the step-by-step process to wrap the text in the text area.
-
Create a class that extends
Application
. -
Create a
TextArea
with the content. -
Set the method
setWrapText()
totrue
for the text area. -
Set the size of
TextArea
. -
Create the
scene
and show it on thestage
. -
The final output will be the text wrapped in the
TextArea
.
Let’s try to implement an example based on the steps above.
package delftstack;
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.control.TextArea;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
public class TextArea_Wrap extends Application {
public void start(Stage TextArea_Stage) {
String Content = "DelftStack is a resource for everyone interested in programming, "
+ "embedded software, and electronics. It covers the programming languages "
+ "like Python, C/C++, C#, and so on in this website's first development stage. "
+ "Open-source hardware also falls in the website's scope, like Arduino, "
+ "Raspberry Pi, and BeagleBone. DelftStack aims to provide tutorials, "
+ "how-to's, and cheat sheets to different levels of developers and hobbyists..";
// Create a Label
TextArea Text_Area = new TextArea(Content);
// wrap the textArea
Text_Area.setWrapText(true);
// Set the maximum width of the textArea
Text_Area.setMaxWidth(300);
// Set the position of the textArea
Text_Area.setTranslateX(30);
Text_Area.setTranslateY(30);
Group TextArea_Root = new Group();
TextArea_Root.getChildren().add(Text_Area);
// Set the stage
Scene TextArea_Scene = new Scene(TextArea_Root, 595, 150, Color.BEIGE);
TextArea_Stage.setTitle("Label Example");
TextArea_Stage.setScene(TextArea_Scene);
TextArea_Stage.show();
}
public static void main(String args[]) {
launch(args);
}
}
The code above will create a text area with the text wrapped in it. See output:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook