How to Center Text in a Pane in JavaFX
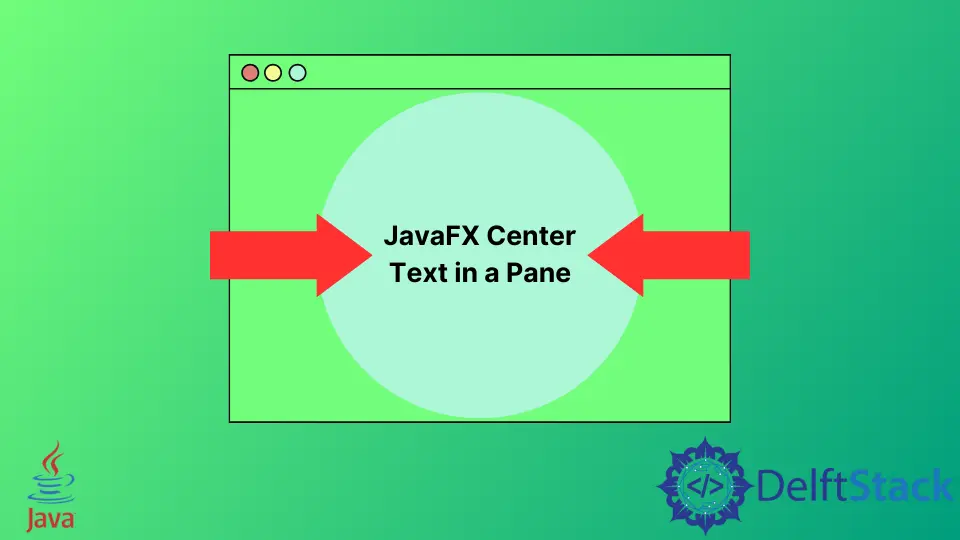
There is no functionality to center the nodes in a Pane
class, but we can use StackPane
if we want to center the nodes. This tutorial demonstrates using a StackPane
to center text or other nodes in JavaFX.
JavaFX Center Text in a Pane
The StackPane
is a kind of pane used to lay out its children to stack up to others. We can align the StackPane
to center the nodes in the pane.
The default alignment property for a StackPane
is Pos.CENTER
. The JavaFX StackPane
is instantiated from JavaFX.scene.layout.StackPane
.
The StackPane
has two constructors used for different purposes. The syntax for StackPane
is:
StackPane Demo = new StackPane();
The constructors of StackPane
are:
StackPane()
: The layout will be created with the defaultPos.CENTER
alignment.StackPane(Nodeā¦. nd)
: The layout will be created with default alignment.
The alignment property can be used to align the nodes in a StackPane
. There are three methods for StackPane
to work with alignment:
getAlignment()
: The method is used to get the alignment property value.setAlignment(Posvalue)
: The method is used to set the alignment property value.setAlignment(Node child, Posvalue)
: The method is used to set the alignment property value for a child node in theStackPane
.
Let’s try an example to center the text of nodes in a pane using the StackPane
. See example:
package delftstack;
import javafx.application.Application;
import javafx.collections.ObservableList;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.layout.StackPane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Circle;
import javafx.scene.shape.Sphere;
import javafx.scene.text.Font;
import javafx.scene.text.FontWeight;
import javafx.scene.text.Text;
import javafx.stage.Stage;
public class JavaFx_StackPane extends Application {
@Override
public void start(Stage DemoStage) {
// Create the text to be centered
Text Demotext = new Text("Centered Text");
// Font for the text
Demotext.setFont(Font.font(null, FontWeight.BOLD, 20));
// color of the text
Demotext.setFill(Color.BLUE);
// position of the text
Demotext.setX(20);
Demotext.setY(50);
// circle
Circle DemoCircle = new Circle(700, 500, 300);
// fill color
DemoCircle.setFill(Color.LIGHTBLUE);
DemoCircle.setStroke(Color.BLACK);
// Now Create a Stackpane
StackPane DemoStackPane = new StackPane();
// Margin for the above circle
DemoStackPane.setMargin(DemoCircle, new Insets(50, 50, 50, 50));
ObservableList li = DemoStackPane.getChildren();
// Add child nodes to the pane
li.addAll(DemoCircle, Demotext);
// Create a scene
Scene DemoScene = new Scene(DemoStackPane);
// Set title
DemoStage.setTitle("Centered Text Sample");
// Add scene to the stage
DemoStage.setScene(DemoScene);
// Display the results
DemoStage.show();
}
public static void main(String args[]) {
launch(args);
}
}
The code creates a text and a circle in the StackPane
and uses the default alignment of the center position. See output:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook