How to Write Byte to File in Java
-
Using
FileOutputStream
to Write Byte to File in Java -
Using
java.nio.file
to Write Byte to File -
Using
Apache Commons IO
Library to Write Byte to File in Java
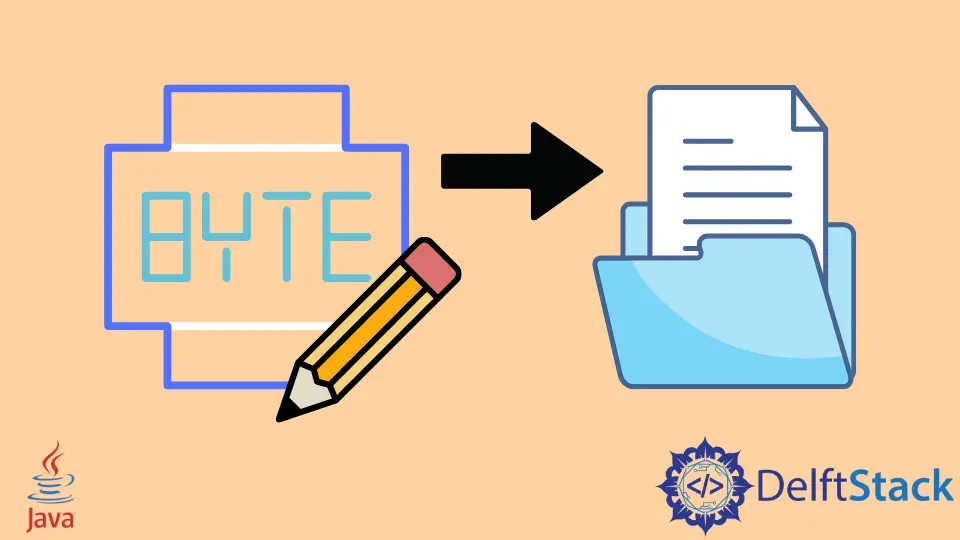
This program demonstrates how to write a byte array to a file in Java. This task can be performed using FileOutputStream
and using some libraries mentioned in this article.
Using FileOutputStream
to Write Byte to File in Java
The Class FileOutputStream
in Java is an output stream used to write data or stream of bytes to a file. The constructor FileOutputStream(File file)
creates a file output stream to write to the file represented by the File
object file
, which we have created in the code below.
The variable s
of type String
is passed to the getBytes()
method, which converts the string into a sequence of bytes and returns an array of bytes. The write()
method takes the byte array as an argument and writes b.length bytes from the byte array b
to this file output stream.
A file with the extension .txt
is created at the given path, and if we open that, we can see the contents same as the string stored in the variable s
.
import java.io.File;
import java.io.FileOutputStream;
public class ByteToFile {
public static void main(String args[]) {
File file = new File("/Users/john/Desktop/demo.txt");
try {
FileOutputStream fout = new FileOutputStream(file);
String s = "Example of Java program to write Bytes using ByteStream.";
byte b[] = s.getBytes();
fout.write(b);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Using java.nio.file
to Write Byte to File
Java NIO ( New I/O)
package consists of static methods that work on files, directories and it mostly works on Path
object. The Path.get()
is a static method that converts a sequence of strings or a path string to a Path. It simply calls FileSystems.getDefault().getPath()
.
Thus, we can write a byte array b
into a file using the Files.write()
method by passing the path to the file, and the byte array converted from a string.
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class ByteToFile {
public static void main(String args[]) {
Path p = Paths.get("/Users/john/Desktop/demo.txt");
try {
String s = "Write byte array to file using java.nio";
byte b[] = s.getBytes();
Files.write(p, b);
} catch (IOException ex) {
System.err.format("I/O Error when copying file");
}
}
}
Using Apache Commons IO
Library to Write Byte to File in Java
The maven dependency for this library is as mentioned below.
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.6</version>
</dependency>
The Apache Commons IO
library has the FilesUtils class, it has writeByteArrayToFile()
method. This method takes the destination path and the binary data we are writing. If our destination directory or file does not exist, they will be created. If the file exists, it will be truncated before writing.
import java.io.File;
import java.io.IOException;
import java.nio.charset.StandardCharsets;
import org.apache.commons.io.FileUtils;
public class ByteToFile {
public static void main(String args[]) {
{
File file = new File("doc.txt");
byte[] data =
"Here, we describe the general principles of photosynthesis"
.getBytes(StandardCharsets.UTF_8);
try {
FileUtils.writeByteArrayToFile(file, data);
System.out.println("Successfully written data to the file");
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
Output:
Successfully written data to the file
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedInRelated Article - Java Byte
- How to Convert Java String Into Byte
- How to Convert Int to Byte in Java
- How to Convert Byte to Int in Java