How to Implement Java while Loop With User Input
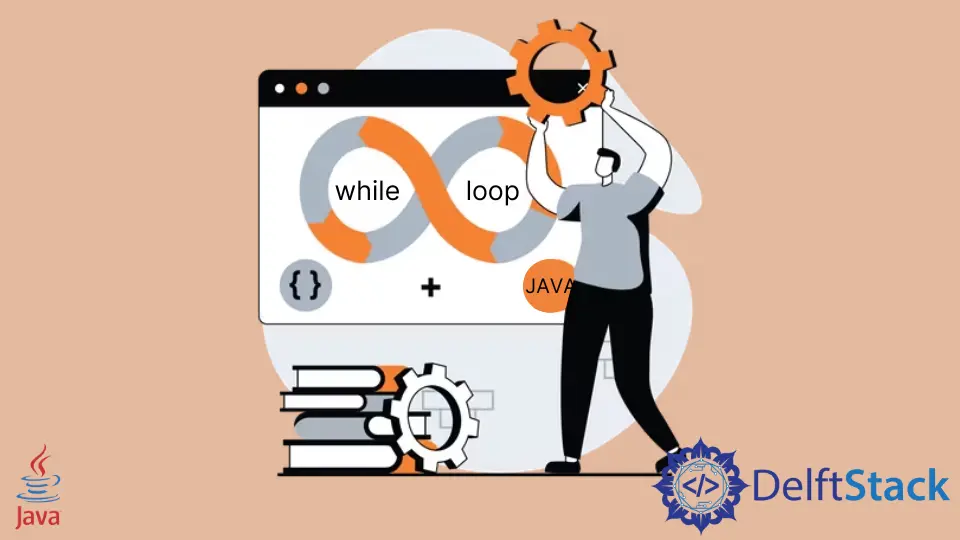
Sometimes, we require multiple user inputs during the loop run while working with loops. This tutorial demonstrates how to create a while
loop that keeps requesting the user input in Java.
Use while
Loop With User Input in Java
We’ll create a while
loop with user input. This example will check if the number is present in the array or not.
The loop will continue to take user inputs till there is an input number that is not a member of the array.
Code:
import java.util.*;
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
int[] input_array = {10, 12, 14, 17, 19, 21};
System.out.println(Arrays.toString(input_array));
System.out.println("Enter number to check if it is a member of the array");
while (true) {
Scanner input_number = new Scanner(System.in);
int number = input_number.nextInt();
if (Arrays.stream(input_array).anyMatch(i -> i == number)) {
System.out.println(number + " is the member of given array");
} else {
System.out.println(number + " is not the member of given array");
System.out.println("The While Loop Breaks Here");
break;
}
}
}
}
Let’s try multiple inputs till the loop breaks. The loop will break when a number is entered which is not a member of the array.
Output:
[10, 12, 14, 17, 19, 21]
Enter number to check if it is a member of the array
10
10 is the member of given array
12
12 is the member of given array
13
13 is not the member of given array
The While Loop Breaks Here
Run code here.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook