Java - Use Nullable Annotation in Method Argument
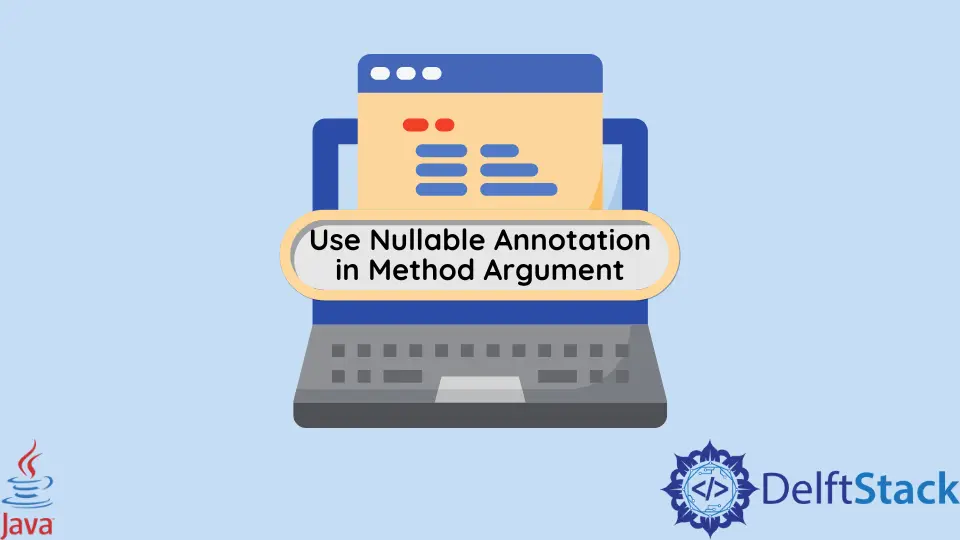
This tutorial briefly discusses the @Nullable
annotation and demonstrates its use in method argument via code example.
@Nullable
Annotation and Its Use in Method Argument
The @Nullable
annotation allows you to create a method call that can return a null
value. Also, you can declare variables that can hold a null
value. It is commonly used to avoid NullPointerExceptions
in Java.
The critical point is that if the parent method contains the @Nullable
, then its child needs to be annotated with @Nullable
. Also, don’t forget to include the package javax.annotation.Nullable
to use @Nullable
; otherwise, you will get an error.
In Java, when you declare a variable or method, initially, it becomes null
, and the annotation @Nullable
makes it more explicit. Now, let’s learn the use of @Nullable
in method arguments using the following code fence.
Example Code:
import javax.annotation.Nullable;
public class JavaNullable {
String printStr(@Nullable String s) {
return s;
}
public static void main(String args[]) {
JavaNullable jnn = new JavaNullable();
System.out.println("The sum is: " + jnn.printStr(null));
}
}
In the example above, we first included the required package for the @Nullable
as import javax.annotation.Nullable;
.
After that, we created a method named printStr()
where we set its argument as @Nullable
, and it will return the result by printing the variable.
Then we created an object of the JavaNullable
class and called its method printStr()
. Now, you will see the output similar to the below when you execute the above code:
The sum is: null
Considering this example, we can say that if we set a method to @Nullable
, it can return null
; similarly, if we set a variable to @Nullable
, it can hold the null
value.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn