How to Create Unsigned Int in Java
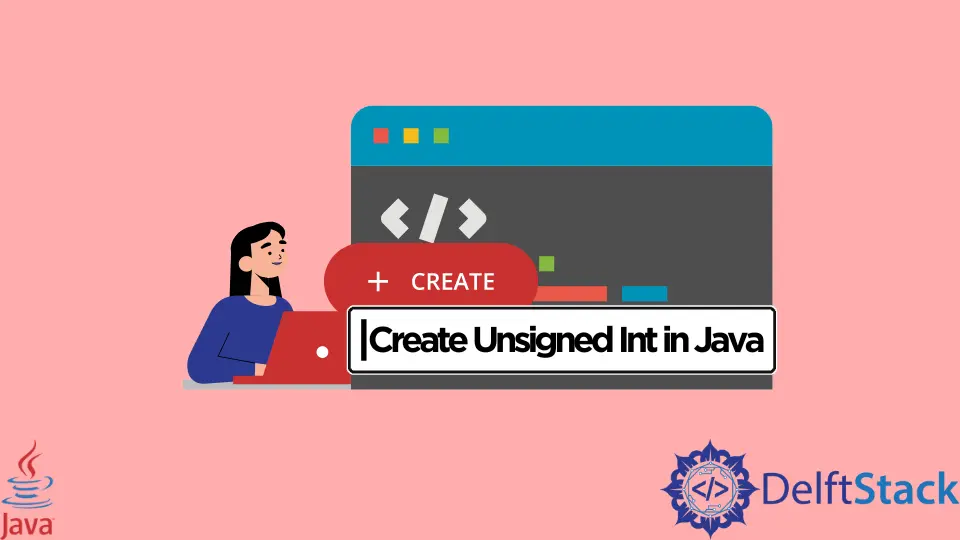
Signed Integers are stored in the database as positive and negative values range, from -1
to -128
. Opposite to that, Unsigned Integers hold the large set of positive range values only, no negative values, from 0
to 255
. It means that unsigned integers can never store negative values.
In Java, the datatype set does not include any unsigned int
explicitly. However, based on the use case, other data types can be treated as unsigned integers. Java provides a long
datatype to store larger unsigned values.
The long
datatype is a 64-bit size and works on two’s-complement internally. In Java 8 and higher versions, the long
datatype can store values ranging from 0
to 2^64-1
. It provides more data range as compared to the simple int
datatype.
Below is the code block to define the size and the difference between the two data types.
public class Main {
public static void main(String[] args) {
System.out.println("Size of int: " + Integer.SIZE + "bits");
System.out.println("Size of int: " + Long.SIZE + "bits");
int signedInt = -2345678;
long unsignedValue = signedInt & 0xffffffffL;
System.out.println(signedInt);
System.out.println(unsignedValue);
}
}
In the code block above, the first two statements print the size of the Integer
and Long
classes. An integer is a primitive datatype to hold int
values.
The size of int
prints to 32 bytes, and that of the Long
wrapper class prints as 64 bytes. The classes have static and final variables defined that represent the size of the wrapper class.
Next to the print statement, two local or class variables are defined. The variable is of the int
and long
data types. The value instantiated in an integer is negative, as it holds both negative and positive values.
In a long
variable, the signed integer is operated with the Bitwise &
operator. It works on two’s complement of a number converted into binary format. So internally, it first converts the negative value into binary form. Between the two binary values, it performs logical &
operations over the two values. The operator will eventually convert the negative value into a positive number.
The output of the two program lines above is shown below.
Size of int: 32bits
Size of int: 64bits
-2345678
4292621618
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedIn