How to Fix the Unexpected Type Error in Java
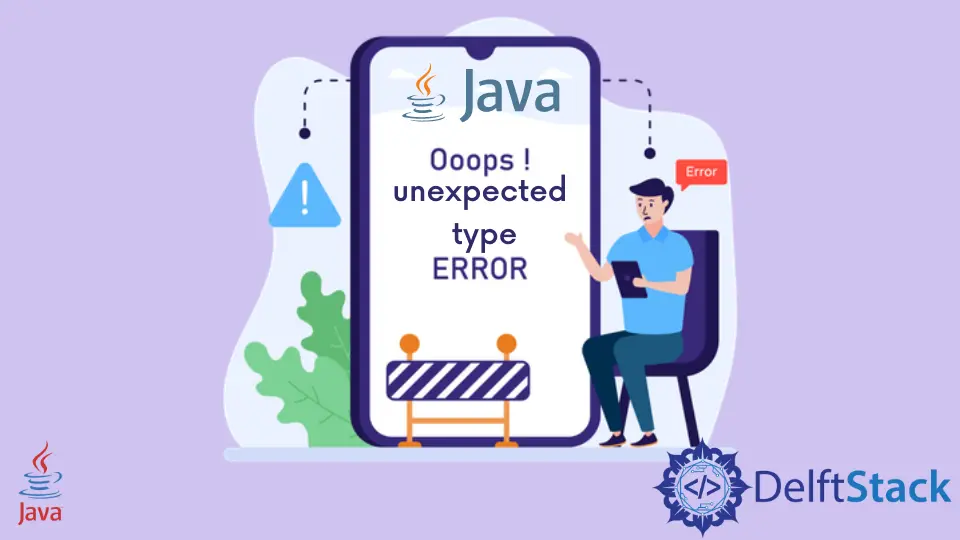
This tutorial demonstrates the unexpected type
error in Java.
Fix the Java unexpected type
Error
The Java unexpected type
error occurs when we try to assign a value to a value or expression, not the variable. This means we can only assign values to variables.
For example, if we try to assign a value to DemoStr.charAt(i)
as it is not a variable but an expression that returns a value and type char, this code will throw the unexpected type
error.
Here is an example of this error:
package delftstack;
public class Example {
public static void main(String[] arg) {
String DemoStr = "delftstack";
String ReversedString = "";
for (int i = DemoStr.length() - 1; i >= 0; i--) {
DemoStr.charAt(i) += ReversedString;
}
System.out.println(ReversedString);
}
}
The code above tries to reverse a string, but it is assigning value to DemoStr.charAt(i)
, which is why it will throw the Java unexpected type
error. See output:
****.java:8: error: unexpected type
required: variable
found: value
The solution for this error can be to assign a value to a variable or use the String buffer and reverse()
method to reverse a string. Here are solutions in both ways:
Solution 1:
package delftstack;
public class Example {
public static void main(String[] arg) {
String DemoStr = "delftstack";
String ReversedString = "";
for (int i = DemoStr.length() - 1; i >= 0; i--) {
ReversedString += DemoStr.charAt(i);
}
System.out.println(ReversedString);
}
}
Solution 2:
package delftstack;
public class Example {
public static void main(String[] arg) {
String DemoStr = "delftstack";
String ReversedString = "";
ReversedString = new StringBuffer(DemoStr).reverse().toString();
System.out.println(ReversedString);
}
}
Both solutions will work similarly with a similar output:
kcatstfled
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn FacebookRelated Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack