How to Get Today's Date in Java
-
Get Today’s Date Using
LocalDate
in Java -
Get Today’s Date Using
Calender
andSimpleDateFormat
in Java -
Get Today’s Date Using
java.sql.Date
in Java
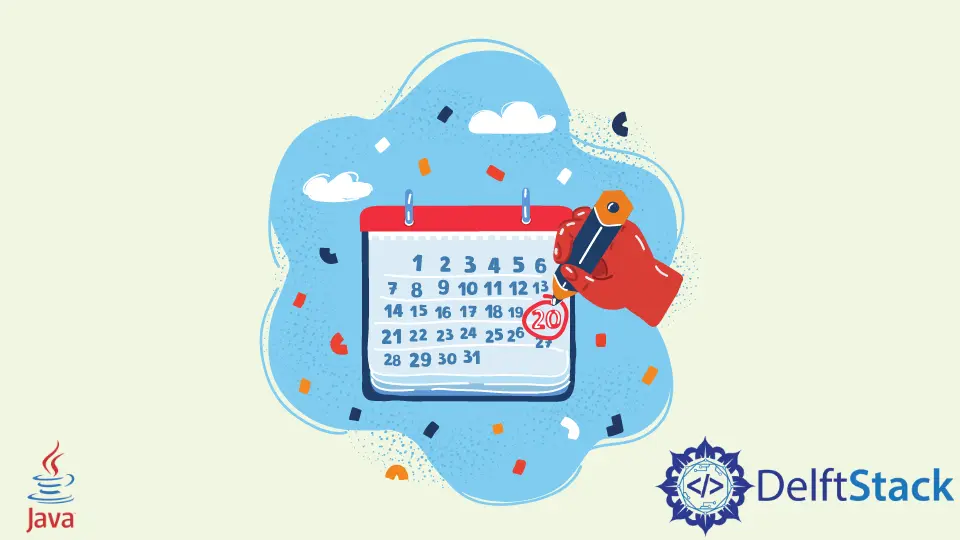
This article introduces methods to get today’s date in Java.
Get Today’s Date Using LocalDate
in Java
LocalDate
is an object that represents a date in the ISO format that is YYYY-MM-DD
. As its name indicates, LocalDate
only holds a date object but not the current time information.
LocalDate.now()
uses the system clock to get the current date in the default time-zone specified in the system. In the output below, we get the date in the ISO format.
import java.time.LocalDate;
public class GetTodayDate {
public static void main(String[] args) {
LocalDate todaysDate = LocalDate.now();
System.out.println(todaysDate);
}
}
Output:
2021-01-03
Get Today’s Date Using Calender
and SimpleDateFormat
in Java
In the second method, we use Calender
to create an object and call the getTime()
method that returns the result as a Date
object. Our goal is to get today’s date, but the result that we get as a Date
tells the current time as well as the time-zone.
We format the dateObj
to get only the date using SimpleDateFormat()
that takes the format that we want as an argument. We use dtf.format()
to format the dateObj
and to get the result as a string.
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
public class GetTodayDate {
public static void main(String[] args) {
SimpleDateFormat dtf = new SimpleDateFormat("yyyy/MM/dd");
Calendar calendar = Calendar.getInstance();
Date dateObj = calendar.getTime();
String formattedDate = dtf.format(dateObj);
System.out.println(formattedDate);
}
}
Output:
2021/01/03
Get Today’s Date Using java.sql.Date
in Java
The last method is useful if we want to work with databases because java.sql.Date
represents the SQL Date that is usually used in databases. The date is calculated as the milliseconds since January 1 1970 00:00:00 GMT. The time part is always set to zero, and it returns only the date.
In the example below, we get the current time in milliseconds and pass it to the constructor of Date
that returns a Date
object. We can see in the output that the date
outputs the date in YYYY-MM-DD
format.
import java.sql.Date;
public class GetTodayDate {
public static void main(String[] args) {
long miliseconds = System.currentTimeMillis();
Date date = new Date(miliseconds);
System.out.println(date);
}
}
Output:
2021-01-03
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn