How to Convert Java Codes to C# Codes
- Understanding the Key Differences Between Java and C#
- Method 1: Manual Conversion
- Method 2: Using Conversion Tools
- Method 3: Leveraging Git for Version Control
- Conclusion
- FAQ
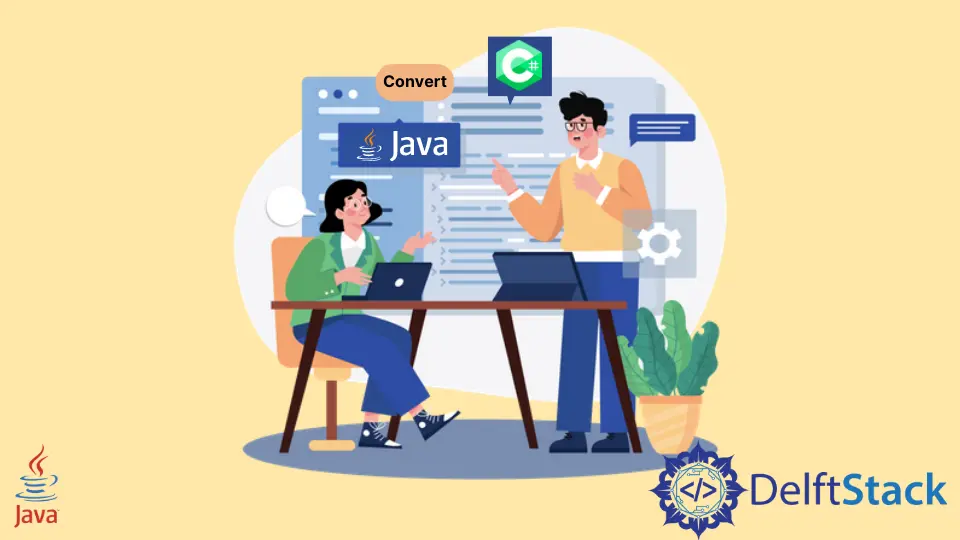
Converting Java code to C# can seem like a daunting task, especially for developers who are accustomed to one language but need to work with the other. Both Java and C# share a lot of similarities, but they also have their unique features and syntax.
In this tutorial, we will explore effective methods to convert Java code to C#. You will learn how to leverage tools and techniques that streamline this process, making it easier for you to transition between these two powerful programming languages. Whether you’re migrating a project or simply looking to understand the differences, this guide will provide you with the insights you need to convert Java code to C# efficiently.
Understanding the Key Differences Between Java and C#
Before diving into the conversion process, it’s essential to understand the key differences between Java and C#. While both languages are object-oriented and share a similar syntax, they have distinct features. For instance, Java runs on the Java Virtual Machine (JVM), while C# operates within the .NET framework. Additionally, C# supports properties and events, which Java does not natively offer. Understanding these differences will help you navigate the conversion process more effectively.
Method 1: Manual Conversion
One of the most straightforward methods to convert Java code to C# is through manual conversion. This approach requires a solid understanding of both languages, allowing you to translate the code line by line.
Here’s a simple example of a Java class and its C# equivalent.
Java Code:
public class Greeting {
public String sayHello(String name) {
return "Hello, " + name + "!";
}
}
C# Code:
public class Greeting {
public string SayHello(string name) {
return "Hello, " + name + "!";
}
}
In the example above, we see a basic class named Greeting
with a method sayHello
in Java. The C# equivalent uses the same class structure but follows C# naming conventions, such as capitalizing method names. The syntax for string concatenation remains similar, making it easier to translate.
Manual conversion allows for a thorough understanding of the underlying logic, but it can be time-consuming, especially for larger codebases. This method is best suited for smaller projects or when you need to ensure that every detail is accurately translated.
Method 2: Using Conversion Tools
For larger projects or when time is of the essence, using automated conversion tools can save you a significant amount of effort. Several online platforms and software can help facilitate this conversion process.
One popular tool is the Java to C# Converter by Tangible Software Solutions. It automates the conversion process and can handle complex code structures.
Here’s how you can use such a tool:
- Copy your Java code.
- Paste it into the converter.
- Click on the convert button.
- Review the generated C# code.
For example, consider the following Java code snippet:
public class Calculator {
public int add(int a, int b) {
return a + b;
}
}
After pasting this code into the converter, you might receive the following C# output:
public class Calculator {
public int Add(int a, int b) {
return a + b;
}
}
Automated tools can handle many of the syntactical differences between Java and C#, such as method naming conventions and type declarations. However, it is crucial to review the output carefully. Automated conversions may not always account for language-specific features or best practices, so manual adjustments may still be necessary.
Method 3: Leveraging Git for Version Control
When converting Java code to C#, using Git can be beneficial for tracking changes and managing versions of your code. This method allows you to maintain a history of your modifications, making it easier to revert if something goes wrong during the conversion process.
Here’s how to use Git effectively during your conversion:
-
Initialize a Git Repository: Start by creating a new Git repository for your C# project.
git init
-
Add Your Java Code: Add your existing Java code to the repository.
git add .
-
Commit the Changes: Commit your Java code to the repository.
git commit -m "Initial commit of Java code"
-
Create a New Branch for C# Conversion: This allows you to work on the C# version without affecting the original Java code.
git checkout -b convert-to-csharp
-
Convert Your Code: As you convert your Java code to C#, make changes and commit them regularly.
git add . git commit -m "Converted Greeting class to C#"
-
Merge Changes: Once you’re satisfied with the C# code, you can merge it back into the main branch.
git checkout main git merge convert-to-csharp
This method not only helps in tracking changes but also allows for collaboration if you’re working in a team. You can easily share your progress and gather feedback, making the conversion process smoother.
Conclusion
Converting Java code to C# doesn’t have to be a daunting task. Whether you choose to do it manually, use automated tools, or leverage Git for version control, understanding the nuances of both languages will make the process much easier. By following the methods outlined in this tutorial, you can ensure a smoother transition between Java and C#. Embrace the challenge, and soon you will find yourself comfortably navigating both languages.
FAQ
-
What is the primary difference between Java and C#?
Java runs on the Java Virtual Machine, while C# is part of the .NET framework, leading to different runtime environments. -
Are there automated tools for converting Java code to C#?
Yes, several online tools can automate the conversion process, such as the Java to C# Converter by Tangible Software Solutions. -
Is manual conversion recommended for large projects?
Manual conversion can be time-consuming for large projects, so using automated tools is often more efficient. -
Can I use Git during the conversion process?
Absolutely! Git is an excellent tool for version control, allowing you to track changes and manage your code effectively. -
Do I need to learn C# to convert Java code?
While understanding C# will help in the conversion process, using automated tools can simplify the task without deep knowledge of the language.
#. Learn effective methods for manual conversion, using automated tools, and leveraging Git for version control. Whether you’re migrating a project or exploring language differences, this guide provides valuable insights to streamline your coding transition.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook