How to Throw Runtime Exception in Java
- Understanding Runtime Exceptions
- Throwing a Runtime Exception
- Custom Runtime Exceptions
- Best Practices for Throwing Runtime Exceptions
- Conclusion
- FAQ
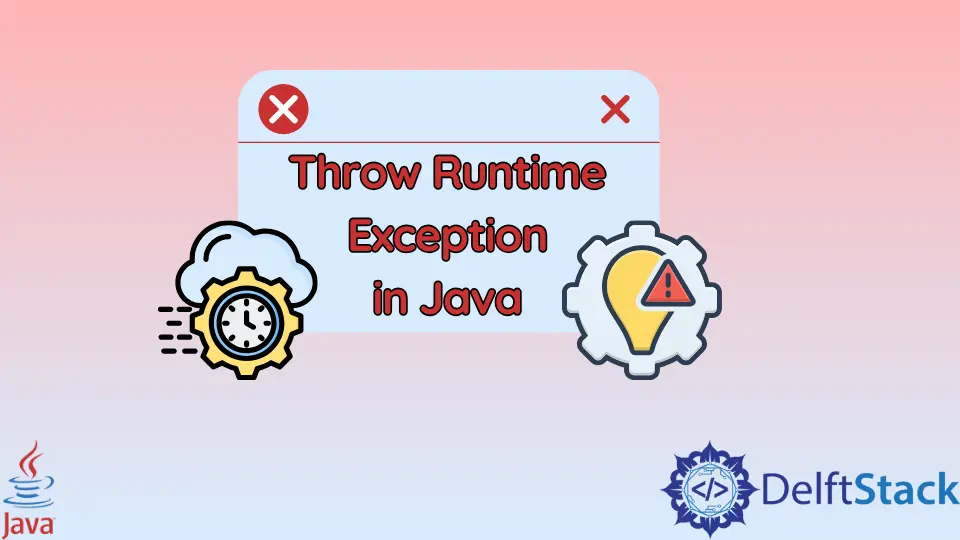
When working with Java, exceptions are a vital part of error handling. Among the various types of exceptions, runtime exceptions are particularly interesting because they indicate problems that can be avoided by the programmer.
In this tutorial, we will explore how to throw runtime exceptions in Java effectively. We will cover the different types of runtime exceptions, when to use them, and provide clear examples to illustrate the concepts. By the end of this guide, you will have a solid understanding of how to manage runtime exceptions in Java, ensuring your applications are robust and error-resistant.
Understanding Runtime Exceptions
Runtime exceptions in Java are unchecked exceptions that can occur during the program’s execution. These exceptions extend the RuntimeException
class and do not need to be declared in a method’s throws
clause. Common examples include NullPointerException
, ArrayIndexOutOfBoundsException
, and IllegalArgumentException
.
The key characteristic of runtime exceptions is that they often indicate programming errors, such as logic flaws or improper use of APIs. Since they can occur at any point during execution, it’s crucial to handle them appropriately to maintain program stability.
Now, let’s dive into how to throw a runtime exception in Java.
Throwing a Runtime Exception
To throw a runtime exception in Java, you can use the throw
statement followed by an instance of a runtime exception class. This can be particularly useful when you want to enforce certain conditions in your code. For example, you might want to throw an IllegalArgumentException
if a method receives an invalid argument.
Here’s a simple example:
public class ExceptionDemo {
public static void checkAge(int age) {
if (age < 18) {
throw new IllegalArgumentException("Age must be 18 or older.");
}
System.out.println("Age is valid.");
}
public static void main(String[] args) {
checkAge(15);
}
}
Output:
Exception in thread "main" java.lang.IllegalArgumentException: Age must be 18 or older.
In this example, the checkAge
method checks if the provided age is less than 18. If it is, it throws an IllegalArgumentException
with a descriptive message. This is a clear way to enforce rules in your application, helping you catch issues early.
Custom Runtime Exceptions
Sometimes, the built-in runtime exceptions do not adequately describe the errors your application might encounter. In such cases, you can create your own custom runtime exceptions by extending the RuntimeException
class. This allows you to provide specific error messages that are relevant to your application’s logic.
Here’s how you can create and throw a custom runtime exception:
public class CustomException extends RuntimeException {
public CustomException(String message) {
super(message);
}
}
public class CustomExceptionDemo {
public static void validateNumber(int number) {
if (number < 0) {
throw new CustomException("Negative numbers are not allowed.");
}
System.out.println("Number is valid.");
}
public static void main(String[] args) {
validateNumber(-5);
}
}
Output:
Exception in thread "main" CustomException: Negative numbers are not allowed.
In this example, we define a CustomException
class that extends RuntimeException
. The validateNumber
method throws this custom exception if the number is negative. This approach provides clarity and specificity to the exceptions thrown by your application.
Best Practices for Throwing Runtime Exceptions
When throwing runtime exceptions, it’s essential to follow best practices to ensure your code remains clean and maintainable. Here are some recommendations:
-
Use Descriptive Messages: Always provide a clear and concise message when throwing an exception. This helps other developers (and your future self) understand the issue quickly.
-
Limit Exception Usage: Only throw runtime exceptions for programming errors that can be avoided by proper coding practices. Avoid using them for recoverable conditions.
-
Document Your Exceptions: If you’re creating custom exceptions, document their purpose and usage in your code. This improves code readability and helps maintainers understand your logic.
-
Avoid Overusing Exceptions: Use exceptions judiciously. Overusing them can lead to performance issues and make your code harder to follow.
By adhering to these best practices, you can effectively manage runtime exceptions in your Java applications, leading to cleaner and more robust code.
Conclusion
Throwing runtime exceptions in Java is a powerful way to handle errors and enforce rules within your applications. By understanding how to use built-in exceptions and create custom ones, you can improve the reliability of your code. Remember to follow best practices to ensure your exceptions are meaningful and easy to manage. With this knowledge, you’re now better equipped to handle runtime exceptions in your Java projects.
FAQ
-
What is a runtime exception in Java?
A runtime exception is an unchecked exception that occurs during the execution of a program, indicating programming errors that can be avoided. -
How do I throw a runtime exception?
You can throw a runtime exception using thethrow
statement followed by an instance of a runtime exception class. -
Can I create my own runtime exception in Java?
Yes, you can create a custom runtime exception by extending theRuntimeException
class. -
What are some common runtime exceptions?
Common runtime exceptions includeNullPointerException
,IllegalArgumentException
, andArrayIndexOutOfBoundsException
. -
Should I catch runtime exceptions?
It’s generally not recommended to catch runtime exceptions unless you have a specific reason to do so, as they often indicate programming errors.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook