How to Throw New Exception in Java
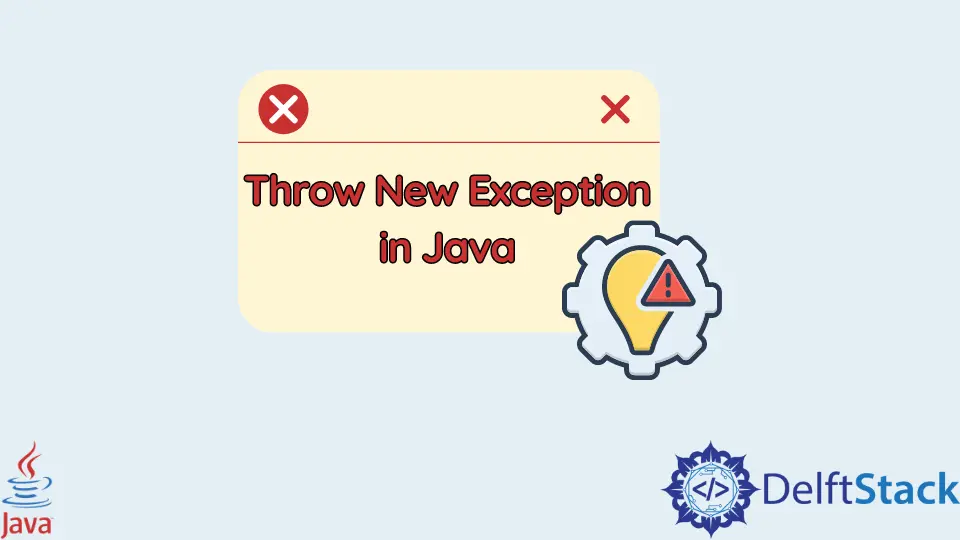
Sometimes we need to show an error message for various purposes when a user runs a program. This message will notify him that the input they provided is not correct.
This article will show how to generate an error using the if ... else
conditional statement. We will also discuss the topic using examples and explanations to make the topic easier.
To show an error message, we need to import a default library of Java called java.lang
.
Generate a Simple Error in Java
Our example below will show a simple error to a user. The code for our example is below.
public class SimpleError {
public static void main(String args[]) {
int a = 40;
if (a == 30)
System.out.println(a);
else
throw new java.lang.Error("This is an error message!!!\n"); // Generating an error
}
}
The above example showed an error message when the variable a
contains a value lower or higher than 30
.
After running the code example above, you will get an output like the one below.
Exception in thread "main" java.lang.Error: This is an error message!!!
at ReplaceStr.main(ReplaceStr.java:5)
Generate a Runtime Error in Java
In this way, we can also generate a runtime error. In our below example, we will show a runtime error when a variable is initiated wrongly.
This example will mostly look like our previous example but has a small difference. The code for our example will be like the below.
public class ReplaceStr {
public static void main(String args[]) {
int a = 40;
if (a == 30)
System.out.println(a);
else
throw new java.lang.RuntimeException("This is an error message!!!\n"); // Generating an error
}
}
The above example showed an error message when the variable a
contains a value lower or higher than 30
.
After running the above code example, you will get an output like the one below.
Exception in thread "main" java.lang.RuntimeException: This is an error message!!!
at ReplaceStr.main(ReplaceStr.java:5)
Please note that the code examples shared here are in Java, and you must install Java on your environment if your system doesn’t contain Java.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn