The ... Parameter in Java
- Using Varargs to Accept Variable Number of Arguments in a Method in Java
- Using Varargs With Multiple Types of Arguments in Java
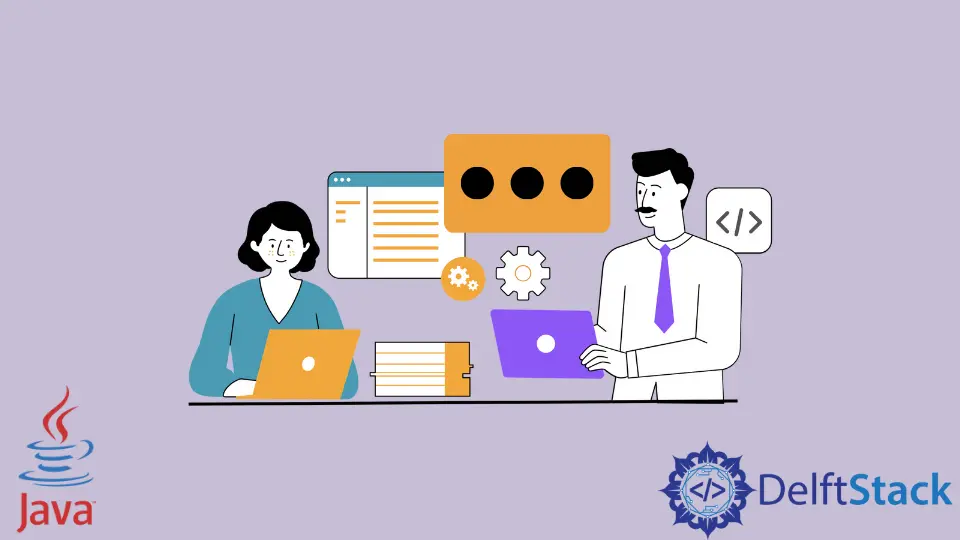
The three dots ...
operator is commonly known as Varargs, which is short for Variable-length Arguments
. Varargs were introduced in JDK 5 to ease using a variable number of arguments. Before this, we had to either use overloading or put the arguments in an array which was not efficient. In the following examples, we will see how we can get the most out of Varargs.
Using Varargs to Accept Variable Number of Arguments in a Method in Java
In this program, we create a static method called functionWithVarArgs()
and set the type of the receiving parameter as String
followed by the three dots and the parameter name str
that means that we can pass any number of String
type arguments.
Inside the functionWithVarArgs()
method, we create an enhanced for
loop that prints every str
value. In the main()
function, we first pass four arguments in the functionWithVarArgs()
function and then call the same function with three arguments again. The output shows that all the passed arguments.
public class Main {
public static void main(String[] args) {
functionWithVarArgs("String 1", "String 2", "String 3", "String 4");
functionWithVarArgs("String A", "String B", "String C");
}
static void functionWithVarArgs(String... str) {
for (String s : str) {
System.out.println(s);
}
}
}
Output:
String 1
String 2
String 3
String 4
String A
String B
String C
Using Varargs With Multiple Types of Arguments in Java
There are a few rules that we have to follow to use the Varargs; the first rule is that the Varargs must be the last argument or the compiler will throw an error, and the second rule is that there cannot be multiple Varargs in a method.
In the following code, we pass two types of arguments, where str
is of type String
and is only a single argument. The last argument of the method functionWithVarArgs()
is the Varargs of type int
. Now in the main()
, we call the function with the first argument as a single value, and the second argument can be of variable length as it is a Vargargs
.
public class Main {
public static void main(String[] args) {
functionWithVarArgs("String 1", 1, 2);
functionWithVarArgs("String A", 10, 20, 30, 40);
}
static void functionWithVarArgs(String str, int... num) {
for (int n : num) {
System.out.println(n);
}
}
}
Output:
1
2
10
20
30
40
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn