Java switch Statement on Strings
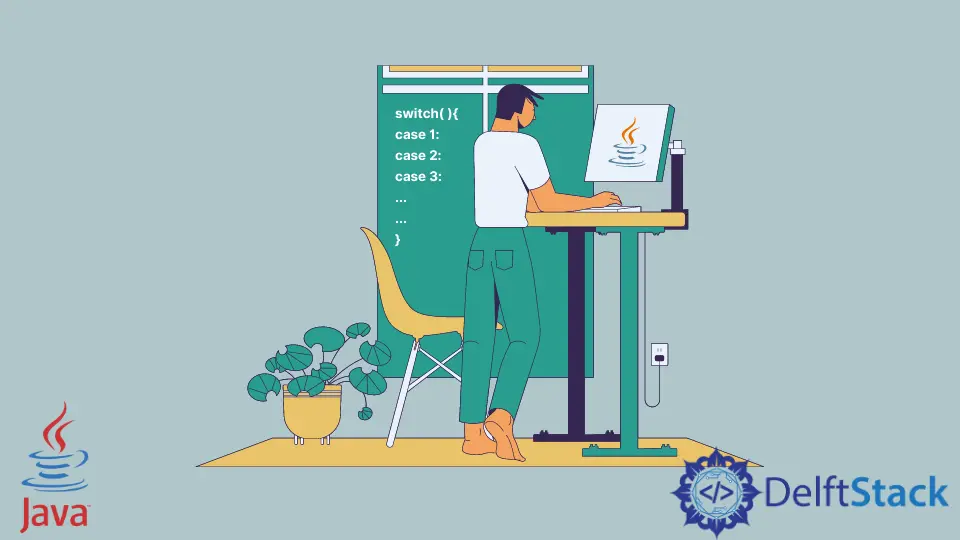
Before JDK 7, it was not possible to use switch
statements on strings, but later on, Java added this functionality. A switch
statement is used for variable equality against a list of values, and these values are called cases
.
This tutorial demonstrates how to use a switch
statement on strings in Java.
Use a switch
Statement on Strings in Java
After JDK 7, we can use a switch
statement on strings in Java, but some important points must be considered.
- The value
switch
must not be null; otherwise, aNullPointerException
will be thrown. - The
switch
statement compares the string based on case sensitivity which means the string in the case and the string passed must be equal with the same case letters. - If the data dealt with is a string, then the values in cases should also be a string type.
Let’s try an example of using switch
statements on strings in Java.
package delftstack;
import java.util.Scanner;
public class Switch_Strings {
public static void main(String args[]) {
Scanner sc = new Scanner(System.in);
System.out.println("Hired Persons at Delftstack: Jack(CEO), John(ProjectManager),"
+ " Tina(HR), Maria(SeniorDeveloper), Mike(JuniorDeveloper), Shawn(Intern)");
System.out.println("Enter the Position of Employee: ");
String Employee_Position = sc.next();
switch (Employee_Position) {
case "CEO":
System.out.println("The Salary of Jack is $ 10000.");
break;
case "ProjectManager":
System.out.println("The Salary of John is $ 8000.");
break;
case "HR":
System.out.println("The Salary of Tina is $ 4000.");
break;
case "SeniorDeveloper":
System.out.println("The Salary of Maria is $ 6000.");
break;
case "JuniorDeveloper":
System.out.println("The Salary of Mike is $ 3000.");
break;
case "Intern":
System.out.println("The Salary of Shawn is $ 1000.");
break;
default:
System.out.println("Please enter the correct position of employee");
break;
}
}
}
The code above uses a switch
statement on a string to print the salary with the name by checking the position. It will ask for user input for the position.
Output:
Hired Persons at Delftstack: Jack(CEO), John(ProjectManager), Tina(HR), Maria(SeniorDeveloper), Mike(JuniorDeveloper), Shawn(Intern)
Enter the Position of Employee:
ProjectManager
The Salary of John is $ 8000.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook