How to Convert String to Timestamp in Java
-
Use
TimeStamp.valueOf()
to Convert a String to Timestamp in Java -
Use
Date.getTime()
to Convert a String to Timestamp in Java -
Convert String Date to
Timestamp
WithTimestamp
Constructor in Java -
Convert Date String to
Timestamp
WithLocalDate
in Java -
Convert String Date to
Timestamp
With theatStartOfDay()
Method in Java
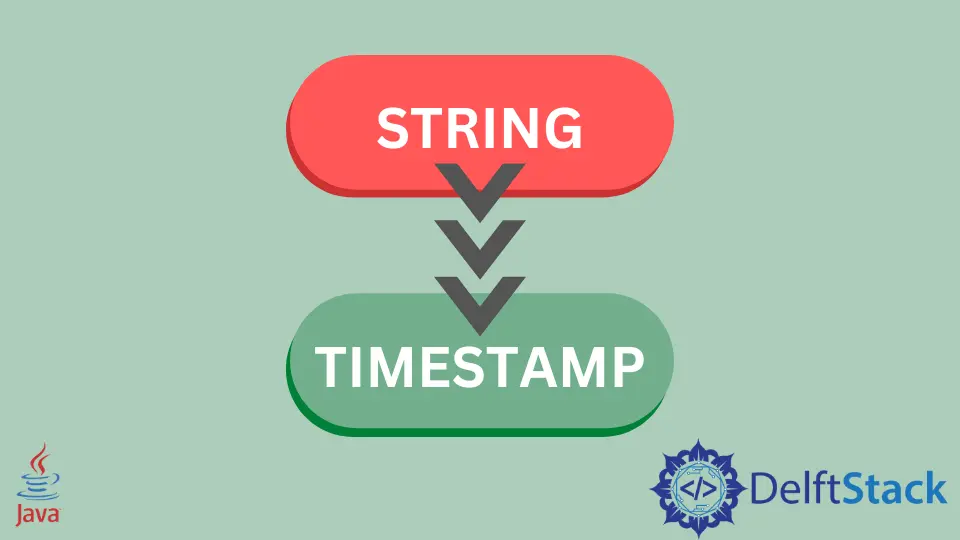
In this article, we will introduce two methods to convert a string to a timestamp in Java. A timestamp is mainly used in databases to represent the exact time of some event. The Timestamp
class we will use in this tutorial is a part of the java.sql.Timestamp
package.
Use TimeStamp.valueOf()
to Convert a String to Timestamp in Java
We will use the TimeStamp
class’s own static function - valueOf()
. It takes a string as an argument and then converts it to a timestamp. One important thing to note here is to take care of the format in which the date and time are written in the string that we want to be converted into a timestamp. It is restricted to a fixed format, which is yyyy-mm-dd hh:mm:ss
.
We cannot change the format and then expect the right result, but instead, if we use an incorrect format, we will get an IllegalArgumentException
in the output. In the below example, we have used 2020-12-12 01:24:23
as the date and time in the string, which follows the correct format of yyyy-mm-dd hh:mm:ss
.
We can now pass dateTime
as the only argument of the valueOf(string)
method, and it will convert a string to a timestamp.
import java.sql.Timestamp;
public class StringToTimeStamp {
public static void main(String[] args) {
String dateTime = "2020-12-12 01:24:23";
Timestamp timestamp = Timestamp.valueOf(dateTime);
System.out.println(timestamp);
}
}
Output:
2020-12-12 01:24:23.0
We can get rid of the date and time formatting restrictions by using the same valueOf()
method, but instead of directly passing a string to the method, we will use the LocalDateTime
class. Because valueOf()
accepts a LocalDateTime
as an argument.
In the following code, dateTime
has a date and time which is then formatted using the DateTimeFormatter
class’s ofPatter()
method. We can use this formatter to parse and get a LocalDateTime
object using the LocalDateTime.from()
function.
Once we get a LocalDateTime
object, we can pass it to Timestamp.valueOf(localDateTime)
to convert the string to a timestamp.
import java.sql.Timestamp;
import java.text.ParseException;
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
public class StringToTimeStamp {
public static void main(String[] args) throws ParseException {
String dateTime = "01/10/2020 06:43:21";
DateTimeFormatter formatDateTime = DateTimeFormatter.ofPattern("dd/MM/yyyy HH:mm:ss");
LocalDateTime localDateTime = LocalDateTime.from(formatDateTime.parse(dateTime));
Timestamp ts = Timestamp.valueOf(localDateTime);
System.out.println(ts);
}
}
Output:
2020-10-01 06:43:21.0
Use Date.getTime()
to Convert a String to Timestamp in Java
The second method to convert a string to a timestamp uses multiple classes and methods. Just like LocalDateTime
, we can use our date and time format in the string. We used the SimpleDateFormate()
class to format the string and then parse it to a Date
object.
We need the Date
object because it has the getTime()
object, which returns the date and time as long
. We can pass this long
value to the constructor of Timestamp
as we have done below.
import java.sql.Timestamp;
import java.text.DateFormat;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
public class StringToTimeStamp {
public static void main(String[] args) throws ParseException {
String inDate = "01/10/2020 06:43:21";
DateFormat df = new SimpleDateFormat("MM/dd/yyyy HH:mm:ss");
Date date = df.parse(inDate);
long time = date.getTime();
Timestamp ts = new Timestamp(time);
System.out.println(ts);
}
}
Output:
2020-01-10 06:43:21.0
Convert String Date to Timestamp
With Timestamp
Constructor in Java
Here, we use the Timestamp
constructor to get a Timestamp
object. First, we use the SimpleDateFormat
class to set the format of date
and then get the date object using the parser()
method, then we use the geteTime()
method to add time with the date and get a timestamp
as a result. See the example below.
import java.sql.Timestamp;
import java.text.DateFormat;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
public class SimpleTesting {
public static void main(String[] args) {
try {
DateFormat dateFormat = new SimpleDateFormat("dd/MM/yyyy");
Date date = dateFormat.parse("12/10/1990");
Timestamp timeStampDate = new Timestamp(date.getTime());
System.out.println(timeStampDate);
} catch (ParseException e) {
System.out.println(e);
}
}
}
Output:
1990-10-12 00:00:00.0
Convert Date String to Timestamp
With LocalDate
in Java
In Java 8, it adds a new java.time
package that contains several classes to deal with date and time. Here, we use the LocalDate
class of that package. We use parse()
method of LocalDate
class to to get LocalDate
object from the date string and then use atTime()
method to get LocalDateTime
object which returns a date with time. The now()
is used to get the current time of the system.
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.time.LocalTime;
public class SimpleTesting {
public static void main(String[] args) {
LocalDate date = LocalDate.parse("2025-11-25");
System.out.println(date);
LocalDateTime dateTime = date.atTime(LocalTime.now());
System.out.println(dateTime);
}
}
Output:
2025-11-25
2025-11-25T09:44:56.814795
Convert String Date to Timestamp
With the atStartOfDay()
Method in Java
The atStartOfDay()
method in LocalDate
class is also use to get LocalDateTime
object. This method adds the time to the date at the start of the day and returns a date-time
rather than a simple date. See the example below.
import java.time.LocalDate;
import java.time.LocalDateTime;
public class SimpleTesting {
public static void main(String[] args) {
String strDate = "2019-10-20";
LocalDate date = LocalDate.parse(strDate);
LocalDateTime dateTime = date.atStartOfDay();
System.out.println(dateTime);
}
}
Output:
2019-10-20T00:00
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn