How to Repeat a String in Java
- Method 1: Using a Loop
- Method 2: Using StringBuilder
- Method 3: Using String.repeat() Method (Java 11 and Above)
- Conclusion
- FAQ
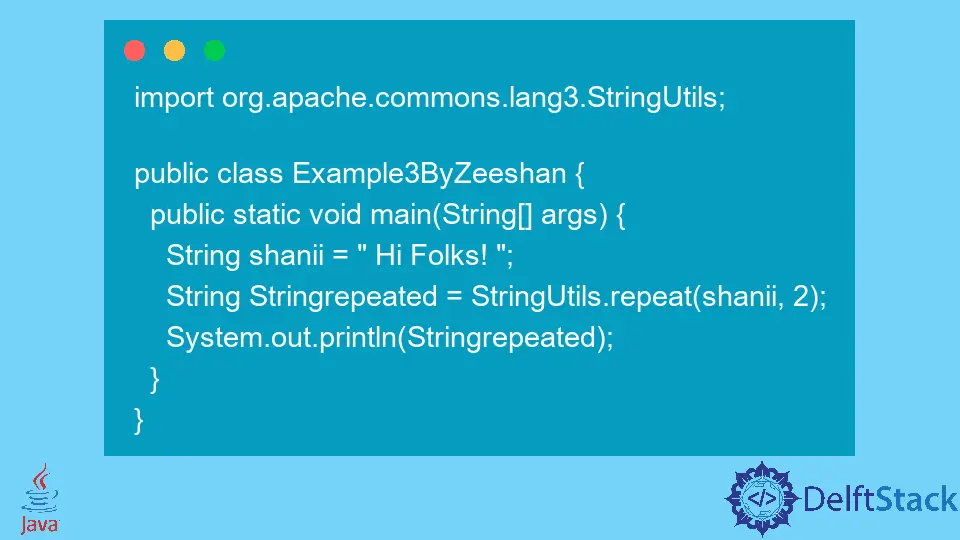
In Java, repeating a string can be a common requirement, whether you’re generating repeated messages, creating patterns, or manipulating text for various applications. Thankfully, Java provides several straightforward methods to achieve this.
In this article, we’ll explore different techniques for repeating a string, including the use of loops, the StringBuilder
class, and the String.repeat()
method introduced in Java 11. Each method will be explained with clear code examples to help you understand how to implement them effectively. Whether you’re a beginner or an experienced developer, you’ll find useful insights here. Let’s dive into the world of string manipulation in Java!
Method 1: Using a Loop
One of the simplest ways to repeat a string in Java is by using a loop. This method involves concatenating the string multiple times based on a specified number of repetitions. Here’s how you can do it:
public class StringRepeater {
public static void main(String[] args) {
String str = "Hello ";
int repeatCount = 3;
String result = "";
for (int i = 0; i < repeatCount; i++) {
result += str;
}
System.out.println(result);
}
}
Output:
Hello Hello Hello
In this example, we declare a string str
and an integer repeatCount
to specify how many times we want to repeat the string. We initialize an empty string result
and use a for
loop to concatenate str
to result
for the number of times defined by repeatCount
. Finally, we print the result. While this method is straightforward, it may not be the most efficient for a large number of repetitions due to the creation of multiple string objects during concatenation.
Method 2: Using StringBuilder
Another effective method for repeating a string is by using the StringBuilder
class. This approach is generally more efficient than using a simple loop because StringBuilder
is designed for mutable strings, reducing the overhead associated with string concatenation. Here’s an example:
public class StringRepeater {
public static void main(String[] args) {
String str = "Hello ";
int repeatCount = 3;
StringBuilder sb = new StringBuilder();
for (int i = 0; i < repeatCount; i++) {
sb.append(str);
}
System.out.println(sb.toString());
}
}
Output:
Hello Hello Hello
In this code snippet, we create a StringBuilder
object called sb
. Inside the loop, we use the append()
method to add str
to sb
for the specified number of repetitions. After the loop, we convert the StringBuilder
back to a string using toString()
and print the result. This method is more memory-efficient, especially when dealing with larger strings or higher repetition counts, making it a preferred choice in many scenarios.
Method 3: Using String.repeat() Method (Java 11 and Above)
If you are using Java 11 or later, you can take advantage of the built-in String.repeat(int count)
method. This method simplifies the process of string repetition significantly. Here’s how you can use it:
public class StringRepeater {
public static void main(String[] args) {
String str = "Hello ";
int repeatCount = 3;
String result = str.repeat(repeatCount);
System.out.println(result);
}
}
Output:
Hello Hello Hello
In this example, we simply call the repeat()
method on the string str
, passing in the number of times we want it to be repeated. This method is not only concise but also highly efficient, as it handles the underlying string manipulation internally. For developers using Java 11 and above, this is the most straightforward and efficient way to repeat strings.
Conclusion
Repeating a string in Java can be accomplished through various methods, each with its own advantages. Whether you choose to use a loop, StringBuilder
, or the String.repeat()
method, it’s essential to consider factors like readability and performance based on your specific use case. For most applications, especially those running on Java 11 or later, the String.repeat()
method is the best choice due to its simplicity and efficiency. By mastering these techniques, you can enhance your string manipulation skills in Java and make your code more effective.
FAQ
- How does the String.repeat() method work in Java?
The String.repeat() method creates a new string by repeating the original string a specified number of times.
-
Is using StringBuilder more efficient than using a loop for string concatenation?
Yes, StringBuilder is generally more efficient because it reduces the overhead of creating multiple string objects during concatenation. -
Can I repeat a string a negative number of times?
No, if you pass a negative number to the String.repeat() method, it will throw an IllegalArgumentException. -
What is the difference between String and StringBuilder in Java?
String is immutable, meaning it cannot be changed once created, while StringBuilder is mutable and allows modifications without creating new objects. -
Which method is the best for repeating strings in Java?
The best method depends on your Java version and specific needs. For Java 11 and above, using String.repeat() is recommended for its simplicity and efficiency.
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn