Comparison Between string.equals() vs == in Java
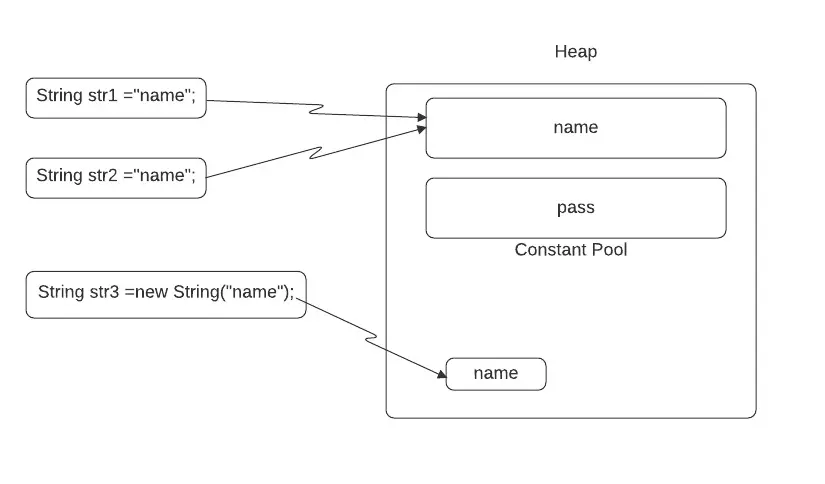
In Java, there are two types of object comparison. The first one is the ==
operator operates on working with the addresses. It internally compares the references of the objects. On the other hand, the equals
method compares the actual content of the instances.
Below is the code block to demonstrate the operators and their behavior.
public class Main {
public static void main(String[] args) {
String str1 = "name";
String str2 = "name";
String str3 = new String("name");
System.out.println(str1.equals(str2));
System.out.println(str1 == str2);
System.out.println(str1.equals(str3));
System.out.println(str3 == str1);
}
}
In the code block above, three instances of string get defined. The string classes are immutable in nature. The immutability means the instance that gets created can never get overridden.
Additionally, the variables hold the memory in the heap location. So while making the string name
, it gets saved in the heap memory. When another new instance, str2
with the same name
value, gets initialized, the JVM does not create another new address for it. Instead, it assigns the reference of the str1
instance to the str2
variable.
The fact holds the proof when we try to compare str1==str2
to both the string instances. The statements results true
as both references point to the same name address in the memory. The fact explained can better be understood in the diagram below.
Similarly, when the equals
method gets applied to both instances, it results in the boolean true
value. As the function works on the content comparison, it is equal.
Next, another string str3
variable gets initialized using the new
keyword. The JVM will internally create a new space in heap memory for the name
address instead of assigning the instance to the str3
variable. So when the content comparison gets made, it returns true
on the equals
function. It returns false
when the address gets compared using ==
operator.
Here is the output of the code block above.
true
true
true
false
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedIn