How to Find a Set Intersection in Java
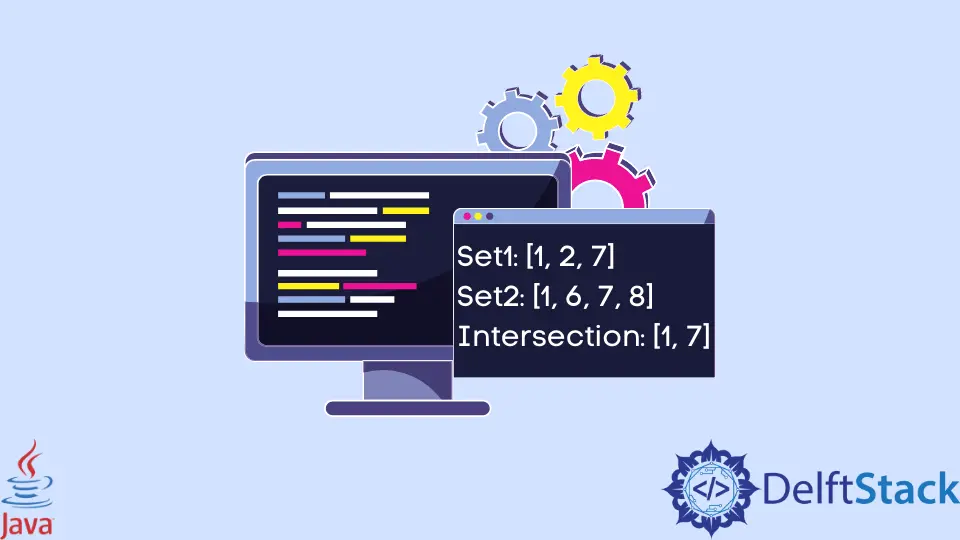
The term Set
is an interface present in the java.util
package. A set is a collection interface that stores unordered lists and does not allow the storage of duplicate entities. Mathematically, the set interface has three properties.
- The elements in the Set are non-null.
- No two elements in the Set can be equal.
- A Set does not preserve insertion order.
Use the Set Insertion and Find the Set Intersection in Java
You can see the program below, which demonstrates the Set insertion and finding the intersection between two sets in Java.
import java.util.Arrays;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
public class SetIntersection {
public static void main(String[] args) {
Set<Integer> s1 = new HashSet<>();
s1.add(2);
s1.add(7);
s1.add(1);
System.out.println("Set1: " + s1);
List list = Arrays.asList(1, 7, 6, 8);
Set<Integer> s2 = new HashSet<>(list);
System.out.println("Set2: " + s2);
Set<Integer> intersection = new HashSet<>(s1);
intersection.retainAll(s2);
System.out.println("Intersection: " + intersection);
}
}
In the code above, a set is declared as the first step of the process. The new HashSet
creates a new instance of the HashSet
class and assigns the reference formed to the Set
instance. The default capacity of HashSet
is 16
, and the load factor is 0.75
. The HashSet
class is compatible with the Set
interface because the HashSet
internally implements the Set interface.
The variable s1
gets initialized using the add
method. The function adds the object of the defined type to the Set instance, considering that the object is non-null and is not duplicate. The function returns boolean
based on whether the value is inserted or not. The function throws a ClassCastException
if the class of the specified element is not similar to that of the Set instance. It throws a NullPointerException
if the element is a null value and an IllegalArgumentException
if some property of the element prohibits its addition into the Set collection.
Another way to create a set is using the list
instance passed into the HashSet
constructor parameter. The list gets initialized with defined values using the asList
method of the Arrays
class. The instance of the list gets passed as a parameter in the HashSet
constructor. The Set collection does not preserve the order in which the elements get stored.
Another instance of the Set gets instantiated with the s1
instance as a constructor parameter. Now, this intersection
reference invokes another method that is the retainAll
function. The function only retains the elements that are present in invoking the instance and the invoked instance. The method returns boolean true
when the Set gets changed in the retain
operation. It throws an UnsupportedOperationException
if it does not support the operation over sets. It throws a ClassCastException
if there are incompatible set types and a NullPointerException
if the Set contains a null element.
The output of the above intersection between sets is as below.
Output:
Set1: [1, 2, 7]
Set2: [1, 6, 7, 8]
Intersection: [1, 7]
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedIn