Java Serial Ports
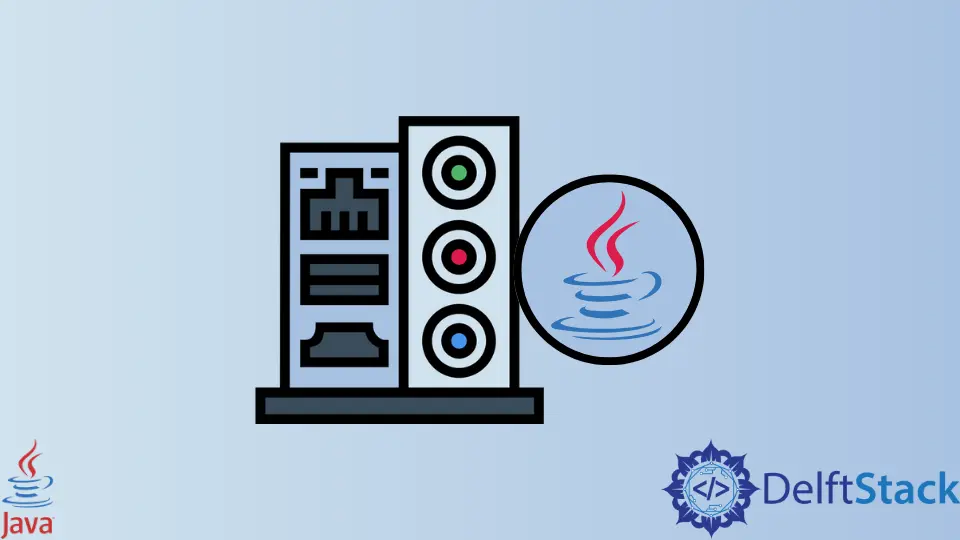
Java Serial Ports are used for serial communication with windows applications. Many options are available to work with serial ports, but most are out of date.
Even the Java API javax.comm
is deprecated in new versions. This tutorial demonstrates how to work with serial ports in Java.
Java Serial Ports Using JSerialComm
API
JSerialComm
is a Java library, which provides a platform-independent way to use serial ports, and we don’t require external libraries. The JSerialComm
is the best alternative to outdated RxTx
and deprecated javax.comm
libraries.
JSerialComm
is easy to use and has enhanced timeouts support. It also supports opening multiple ports simultaneously.
Follow the steps to use the serial ports in Java.
-
First of all, download
JSerialComm
. -
Add the
jar
file to your build path, and then you are ready to useJSerialComm
.
Let’s try a simple example to print the serial ports on our device. It should be mentioned here that if your device doesn’t have ports, the code will not print any port.
See example.
package delftstack;
import com.fazecast.jSerialComm.SerialPort;
public class Serial_Port {
public static void main(String[] args) {
System.out.println("Hello this is a java program to check ports from delftstack");
SerialPort[] Device_Ports = SerialPort.getCommPorts();
for (SerialPort each_port : Device_Ports) {
System.out.println(each_port.getSystemPortName());
}
}
}
It doesn’t show any ports for our system because it doesn’t have any ports, shown from the output and the device manager screenshot.
Hello this is a java program to check ports from delftstack
This link also provides a Java project in a zip file that can be imported. This project has a test program that shows almost all the operations of serial ports.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook