How to Run Command Line in Java
- Using ProcessBuilder to Run Command Line in Java
- Using Runtime.getRuntime().exec() to Run Command Line in Java
- Conclusion
- FAQ
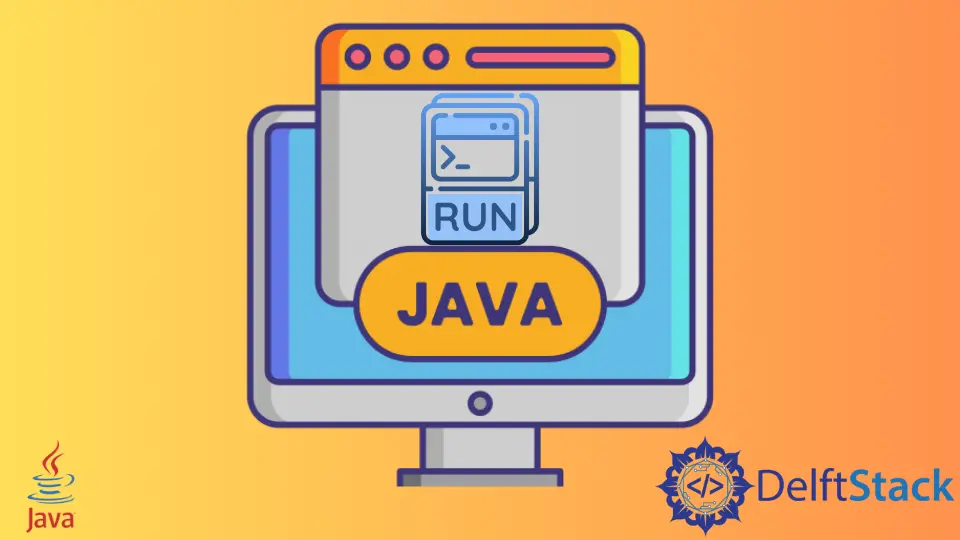
Running command line commands from Java can be a powerful way to enhance your applications. Whether you’re looking to automate tasks, manage files, or integrate with other tools, executing command line instructions can provide a seamless experience.
In this article, we will explore two primary methods for executing command line commands in Java: using the ProcessBuilder and the Runtime.getRuntime().exec() method. We will focus specifically on how these methods can be applied to Git commands, allowing you to perform version control operations directly from your Java applications. By the end of this article, you’ll have a clear understanding of how to run command line operations in Java effectively.
Using ProcessBuilder to Run Command Line in Java
The ProcessBuilder class is a flexible way to create and manage operating system processes. It allows you to start a process with specific attributes and configurations. Here’s how you can use ProcessBuilder to execute Git commands in Java.
import java.io.BufferedReader;
import java.io.InputStreamReader;
public class GitCommandUsingProcessBuilder {
public static void main(String[] args) {
try {
ProcessBuilder processBuilder = new ProcessBuilder("git", "status");
processBuilder.redirectErrorStream(true);
Process process = processBuilder.start();
BufferedReader reader = new BufferedReader(new InputStreamReader(process.getInputStream()));
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
process.waitFor();
} catch (Exception e) {
e.printStackTrace();
}
}
}
Output:
On branch master
Your branch is up to date with 'origin/master'.
nothing to commit, working tree clean
The above code demonstrates how to use ProcessBuilder to run the git status
command. First, we create a new instance of ProcessBuilder, passing in the command and its arguments as a list. We then redirect the error stream to the standard output stream for easier reading. The start()
method is invoked to begin the process, and we read the output using a BufferedReader. Finally, we wait for the process to complete using waitFor()
. This method is particularly useful for running multiple commands in a single process, as it allows for greater control over the execution environment.
Using Runtime.getRuntime().exec() to Run Command Line in Java
Another way to execute command line commands in Java is by using the Runtime class’s exec() method. This method is straightforward and allows you to run commands directly. Here’s how you can use it to execute Git commands.
import java.io.BufferedReader;
import java.io.InputStreamReader;
public class GitCommandUsingRuntime {
public static void main(String[] args) {
try {
Process process = Runtime.getRuntime().exec("git status");
BufferedReader reader = new BufferedReader(new InputStreamReader(process.getInputStream()));
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
process.waitFor();
} catch (Exception e) {
e.printStackTrace();
}
}
}
Output:
On branch master
Your branch is up to date with 'origin/master'.
nothing to commit, working tree clean
In this example, we use Runtime.getRuntime().exec()
to execute the git status
command. Similar to the previous method, we read the output using a BufferedReader. The exec()
method creates a new process for the command, allowing us to capture its output. However, it’s essential to handle exceptions properly, as the exec() method can throw various exceptions, such as IOException. While this method is simpler than ProcessBuilder, it lacks some of the advanced features, such as process redirection.
Conclusion
Running command line commands in Java can significantly enhance the functionality of your applications, especially when it comes to version control with Git. By using either ProcessBuilder or Runtime.getRuntime().exec(), you can easily integrate command line operations into your Java code. Each method has its advantages, so choose the one that best fits your needs. With these techniques, you can automate tasks, streamline workflows, and improve your application’s overall performance.
FAQ
- What is the difference between ProcessBuilder and Runtime.exec()?
ProcessBuilder offers more flexibility and control over the process environment, while Runtime.exec() is simpler and more straightforward for quick command execution.
-
Can I run multiple Git commands using ProcessBuilder?
Yes, you can chain commands together in ProcessBuilder by using a shell command that allows for command chaining, like&&
. -
How do I handle errors when running commands in Java?
You can redirect the error stream to the output stream using ProcessBuilder or read from the error stream of the Process object in both methods. -
Is it necessary to wait for the process to finish?
Yes, usingwaitFor()
is essential to ensure that the main thread waits for the command to complete before proceeding. -
Can I run commands that require user input?
Yes, but you will need to handle input streams properly to send user input to the process.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook